How to Disable Chrome notifications in Selenium WebDriver
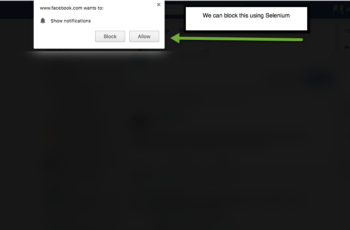
10
Oct
I am sure that you might have faced notification bar in Selenium while working with Chrome Browser. In this article, I will guide you how to Disable Chrome notifications Selenium webdriver.
In previous, post we also discussed how to disable developer option mode in Selenium.
If you have not faced any scenario like this then check below screenshot to check how it looks.
Program to Disable Chrome notifications Selenium Webdriver
import java.util.HashMap; import java.util.Map; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; public class HandlePopup { public static void main(String[] args) throws Exception { // Create object of HashMap Class Map<String, Object> prefs = new HashMap<String, Object>(); // Set the notification setting it will override the default setting prefs.put("profile.default_content_setting_values.notifications", 2); // Create object of ChromeOption class ChromeOptions options = new ChromeOptions(); // Set the experimental option options.setExperimentalOption("prefs", prefs); // pass the options object in Chrome driver WebDriver driver = new ChromeDriver(options); driver.get("https://www.facebook.com/"); driver.manage().window().maximize(); driver.findElement(By.id("email")).sendKeys("urid"); driver.findElement(By.id("pass")).sendKeys("urpass"); driver.findElement(By.id("loginbutton")).click(); } }
I hope this post might help if yes then kindly share with your friends.
Hello Mukesh ,
Thanks for your amazing videos that are helping me to solve many issues in automation , i just need to know how to deal the onesignal slidedown that we get on many website stating”We’d like to send you notifications for the latest news and updates.” allow and cancel button. using selenium !
Hi Risha,
You need to use ChromeOptions to deal with it as mentioned here chromeOptionsObj.addArguments(“–disable-notifications”);
Hi Mukesh
How to disable geolocation popup blockers in firefox browsers . I am unable to use alert functions in webdriver io to disable and enable location pop ups.
Hi Ramana,
Use FirefoxOptions as mentioned below
FirefoxOptions option = new FirefoxOptions();
option.addPreference(“geo.enabled”, false);
and let me know with your observations
Hi Mukesh,
Thanks for your continuous support. I stuck with an issue ,where my scripts are running fine in local machine but when i tried to run through Jenkins(our Vm’s are located in a different location(AWS)) it is failing to navigate to my homepage.Error is Unable to find element . It is giving that error in Production node whereas when i am running those in UAT3 through Jenkins my Script is wkng fine.
Hi Sunil,
Just want to know that is there any SSO/other auth type required to login into application because I’ve seen this scenario some applications. Usually in local env, SSO works and no need to take care of login page but while executing on remote machines, it requires username and password as authentication.
Hi Mukesh,Unable to select Drop down ajax value selenium webdriver .can you please tel me the way of select drop down box when it is in ajax script
Hi Pradeep,
Probably it might not have select tag so in this case, please refer this link for idea to implement workaround.
Hi mukesh,i have written selenium script for facebook login .after login face book account then problem occur allow notifications .how to close allow notifications in Firefox browser .let me know the solution please
Hi Pradeep,
I hope that you went through my post carefully, isn’t? Because I also took same fb login example.
Hi Mukesh, All the solutions available online are for scenario where user gets notification as soon as webpage appears. In my case, I want to handle notification after opening webpage and performing many steps. I am actually clicking on record audio button on the page and then I need to allow. Help would be much appreciated. Thanks!!
Hi Meet,
As of now, Selenium doesn’t allow to notofication pop up as per your requirement beacuse these kind of notification pop up doeosn’t come on Web UI. You can look chromeoptions for allow option for notification pop up.
Hello
How do you block geolocation?
Hi Brian,
Try with this chrome option options.addArguments(“–disable-geolocation”) and please reply back.
Hi Mukesh,
In interviews they demand each and every line explanation of the code.And also i cannot understand the code.Can u please explain the code that u have written??
Hi Shambo,
It is better if you do some project work in order to automate a web application. Then only you will gain some knowledge and feel confident.
Hello Mukesh,
Can you please explain me “options.setExperimentalOption(“prefs”, prefs);”
Hi Natasha,
Using this we can change/set default behavior of chrome browser. Set as many options you want and put them in hashmap. Later on using above statement, set all those options in one shot.
thank you, Mukesh.
Hi Natasha,
Thanks for your reply comments
Hi Mukesh
When I was execute my code the below error displayed some time.
Element is not currently visible and so may not be interacted with (WARNING: The server did not provide any stacktrace information)
Command duration or timeout: 9 milliseconds
Build info: version: ‘2.53.1’, revision: ‘a36b8b1’,
System info:os.name: ‘Windows 7’, os.arch: ‘x86’, os.version: ‘6.1’, java.version: ‘1.8.0_111’
Driver info: org.openqa.selenium.firefox.FirefoxDriver
Capabilities [{applicationCacheEnabled=true, rotatable=false, handlesAlerts=true, databaseEnabled=true, version=47.0.1,
platform=WINDOWS, nativeEvents=false, acceptSslCerts=true, webStorageEnabled=true, locationContextEnabled=true,
browserName=firefox, takesScreenshot=true, javascriptEnabled=true, cssSelectorsEnabled=true}]
Hi Rajesh,
Kindly follow below guide
https://vistasadprojects.com/mukeshotwani-blogs-v2/solve-elementnotvisibleexception-in-selenium-webdriver/
Hi Mukesh,
Can you please explain the line: prefs.put(“profile.default_content_setting_values.notifications”, 2);
I mean what does the number 2 in the above line specifies ?
0- Default
1- Allow
2- Block
I want to allow the popup & for that I am setting it to 1, but it didn’t work for me.
Is there any other solution for this?
Hi Sanjana,
Could you please try without any chrome options and let me know with observartions.
Hi Mukesh sir Plz in the code chrome driver path is missing.so,it’s coming some error
HI Manju,
Its not missing I am using MAC so I did below setting so I don’t have to mention path of chrome again and again https://vistasadprojects.com/mukeshotwani-blogs-v2/chrome-browser-on-mac-using-selenium/
Hi Mukesh! Hope you are doing great!
I have 1 query related to the Sikuli. 1 of my colleagues has used this method to drag and drop a file from the local folder in the system to the application. But he has used the image as path and then later gave the commands such as driver.doubleclick(..); but I want to know it from you clearly on how to use this method. Kindly guide me to the scenario where it is required for us to drag a file from a local folder to the web application. also please let me know other uses of Sikuli and also how we configure it.
Hi Fareed, sure I will make one post on this.