How to execute Selenium Webdriver in Chrome Browser
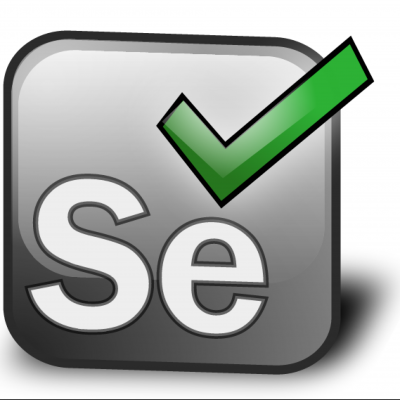
Hello Welcome to Selenium Tutorial, today we will discuss Launch Chrome Browser using Selenium Webdriver.
Selenium Webdriver by default support firefox browser only that is the reason we did not face any issue while working with Firefox.In order to execute your script in the different browser like chrome, IE etc.
If you are working with IE browser then you should know the challenges as well which you will face while working with IE browser. This is one of the most important question in interviews as well.
We have to download separate drivers and we have to specify the path as well with the location of the driver.
Note- Selenium Webdriver supports chrome latest version.
You can refer complete YouTube video
Step 1: Download Chrome Drive
Open any browser and open http://www.seleniumhq.org/download/
Here you will get third party driver section and you can get all the external driver for different browsers.
Click on 2.22 link
Note- Latest chrome version is 2.22 so you will get the latest version
Here you will get the driver zip file which you can extract, after extraction, you will get chromedrive.exe file
Note- Selenium provides only 32 bit but you can use the same for 64-bit machines as well. Practically I tried and it works fine
Program for Run Selenium Webdriver in chrome Browser
package demotc; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.testng.annotations.Test; public class Openchrome { @Test public void test12() throws Exception{ // Initialize browser WebDriver driver=new ChromeDriver(); // Open Google driver.get("http://www.google.com"); // Close browser driver.close(); } }
Output:-
Your test case will fail and you will get IllegalStateException which says we need to specify the chrome driver path where it resides.
If you notice Selenium also gives a very meaningful message that we need to add some chrome variable also while running the script.
Variable name is – webdriver.chrome.driver
In Java to set variable we use setProperty method of System class so let us add the same in our program
Sample Program for Launch Chrome Browser using Selenium Webdriver
import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class TestChrome { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "path of the exe file\\chromedriver.exe"); // Initialize browser WebDriver driver=new ChromeDriver(); // Open facebook driver.get("http://www.facebook.com"); // Maximize browser driver.manage().window().maximize(); } }
Thanks for visiting my blog. Keep reading. Have a nice day 🙂
public static void main(String[] args) throws InterruptedException
{
System.setProperty(“webdriver.chrome.driver”,”C:\\Users\\Pradeep\\Downloads\\Selenium Driverch\\chromedriver.exe”);
WebDriver driver=new ChromeDriver();
driver.get(“https://www.facebook.com/”);
driver.close();
}
}
But facing same issue
error occurred during initialization of boot layer
java.lang.module.FindException: Module format not recognized: C:\Users\Pradeep\Downloads\Selenium Driverch\chromedriver_win32.zip
Hi Pradeep,
Please check whether chromedriver being given is correct or not. Enable Show File extensions in Windows explorer settings and reconfirm file path
Hello Mukesh !
I am not getting the “import( from openqa…..)” option when I hover the mouse over WebDriver.
Hope you can help.
Thanks!
Hi Shaheen,
Check whether you are able to see Selenium dependency into the project build path. Also, there shouldn’t any duplication for the same under build path.
Exception in thread “main” java.lang.IllegalStateException: The driver executable does not exist: C:\Users\sourav\Desktop\chromedriver_win32.exe
i am getting this; error
Hi Priyanka,
Kindly check whether you have given the correct file path and key as “webdriver.chrome.driver” because this is case sensitive
Hi,
I am getting below error ;
Exception in thread “main” java.lang.IllegalStateException: The driver executable is a directory: C:\Users\hp\Downloads\chromedriver_win32 (1)
Hi Nidhi,
Path which you have given C:\Users\hp\Downloads\chromedriver_win32 (1) is not driver.exe path. Kindly check it again
Hello Mukesh,
First of all thanks for the tutorials, your contents are easy to understand.
I just installed the most recent version of Eclipse and added the Jars.
I tried launching Chrome and Firefox Browsers and getting following error with both.
Error: Unable to initialize main class ChromeBrowser.LaunchChrome
Caused by: java.lang.NoClassDefFoundError: org/openqa/selenium/WebDriver.
Thanks,
Ritu
Hi Ritu,
Hope you are using Java 8, if not the install it
Hi, Good day,
I’ve got the foolowing message:
Starting ChromeDriver 80.0.3987.16 (320f6526c1632ad4f205ebce69b99a062ed78647-refs/branch-heads/3987@{#185}) on port 3438
Only local connections are allowed.
Please protect ports used by ChromeDriver and related test frameworks to prevent access by malicious code.
Jan 06, 2020 12:47:35 PM org.openqa.selenium.remote.ProtocolHandshake createSession
INFO: Attempting bi-dialect session, assuming Postel’s Law holds true on the remote end
Jan 06, 2020 12:47:36 PM org.openqa.selenium.remote.ProtocolHandshake createSession
INFO: Falling back to original OSS JSON Wire Protocol.
Jan 06, 2020 12:47:37 PM org.openqa.selenium.remote.ProtocolHandshake createSession
INFO: Detected dialect: OSS
I had tried to open Google or other different browsers, it open a blank browser, but then immediately closes.
I have installed jdk1.8.0_231 and selenium-server-standalone-3.0.1.jar.
What do you think?
Hi Alejandro,
Kindly check whether your chrome browser is also on the same version(i.e. 80.0.3987.16) as mentioned above.
Moreover, could you please try with Selenium 3.141.59.
Tuve el mismo problema y se debía a que la versión del ChromeDriver (v84) era más actual que la versión de mi navegador Google Chrome (v80).
Solución: Actualicé el navegador Google Chrome.
Hi Elias,
Kindly mention in English so that everybody can understand your thoughts…:)
sir, from past 10 days i tried different websites scripts to launch a browser it did not work finally it worked with your simple srcipt thank u for this
You’re welcome lalitesh…
Feel free to drop your doubts in comment section…:)
Hi , What code I have write here when i need to ask user to enter URL manually instead of directly passing in csript
Hi Shalu,
There are multiple ways to handle this requirement. As it looks interactive then you user has to pass url in runtime through cmd/terminal.
If you are using maven project then using surefire plugin, we can pass url value as parameter. Other way is to provide url as parameter from terminal which should be accepted by java main class as arguments later on passed to your actual script.
Hi Mukesh,
when i executed the above code ,I am getting the below error even though I mentioned the correct path of chrome driver.
Exception in thread “main” java.lang.IllegalStateException: The driver executable does not exist:
can you please let me know why I am getting this.
Thank you.
Hi Revathy,
I hope you have mentioned full path of driver executable which includes extension too…
After watching your YouTube video I resolved my mistake and it’s working fine.
Thank you for the response.
Hi Revathy,
Great…:)
Thanks..Now it’s working.
Cheers…!!!
Thanks Mukesh for your prompt response.
I’ll check and update you in case of any issues
Sure…:)
Hi Mukesh,
While executing below code I am getting below mentioned error message
Error: Unable to initialize main class ChromeBrowser.LaunchApplication
Caused by: java.lang.NoClassDefFoundError: org/openqa/selenium/WebDriver
Currently I have installed Java 1.9.0.4 along with “selenium-java-3.141.59” version.
Kindly suggest if any alternative solution for the same.
Immediate response would be highly appreciated.
Hi Lakshmi,
Better uninstall JDK 9 -> Restart your machine -> Install JDK 8 -> Then run your code
Thank you so much Mukesh Otwani.
It’s working now, don’t get tired of helping us…GOD Bless You 🙂
Hi Jibay,
You’re welcome…:)
Hi,
I’m having this error, dunno what to do I’m beginner in Selenium.
Thank you for the help,
Chrome Version : Version 75.0.3770.100 (Official Build) (64-bit)
– this is the drive that i download for my version
-If you are using Chrome version 75, please download ChromeDriver 75.0.3770.90
Java Version : jdk-12.0.1_windows-x64_bin
—–
Exception in thread “main” java.lang.Error: Unresolved compilation problems:
WebDriver cannot be resolved to a type
ChromeDriver cannot be resolved to a type
By cannot be resolved
By cannot be resolved
at co.jibz.selenium.demo/co.jibz.selenium.demo.Demo.main(Demo.java:11)
Hi Jibay,
It would be better if you use JDK 8. Steps are below
1. Uninstall Java 12
2. Restart your computer(for better safety)
3. Install JDK 8, latest version
And run your script
Hi,
I have receiving this error,
the type org.openqa.selenium.chrome.chromedriver is not accessible
how do i fix this?
Hi Prakash,
Which version of java are you using?
Hi sir,
I’m not able to launch chrome using selenium.
public class Demo {
public static void main(String[] args) {
System.setProperty(“webdriver.chrome.driver”, “C:\\Users\\WIN8\\chromedriver.exe”);
WebDriver driver = ChromeDriver(); //showing error in this line
driver.get(“http://google.com”);
}
}
ERROR :
Exception in thread “main” java.lang.Error: Unresolved compilation problem:
The method ChromeDriver() is undefined for the type Demo
at intro.Demo.main(Demo.java:14)
Hi Venkat,
You have written wrong way to initialize ChromeDriver object. Write it as WebDriver driver = new ChromeDriver();
TQ for ur response sir
You’re welcome…:)
Hi
this is swami My browser opening through the code but in google required URL not opened.
its showing blank.
Hi Swamy,
Assuming that your code is good, are you using compatible version of chromedriver with Chrome browser version. If not, kindly check this link http://chromedriver.chromium.org/downloads
hi sir i am not able to launch this program Version 75.0.3770.80 (Official Build) (64-bit)
Hi Priya,
What exception/erro message are you getting while launching Chrome browser through Selenium? Also mention which Chrome browser and ChromeDriver version you used.
Hi I am not able to launch Chrome using above code and getting below error
org.openqa.selenium.os.OsProcess checkForError
SEVERE: org.apache.commons.exec.ExecuteException: Process exited with an error: 1 (Exit value: 1)
Hi Milind,
Kindly mention which version of Chrome browser & Chrome Driver are you using ?
Hi,
Thanks for the detailed explanation. I have downloaded chrome exe file in my PC and given the path in program. But it shows the below error, pls correct me:
“Starting ChromeDriver 74.0.3729.6 (255758eccf3d244491b8a1317aa76e1ce10d57e9-refs/branch-heads/3729@{#29}) on port 32753
Only local connections are allowed.
Please protect ports used by ChromeDriver and related test frameworks to prevent access by malicious code.”
Hi Rans,
These are not error but chromedriver logs which are usual while launching Chrome browser. Just want to know that whether Chrome launched or not?
Hey Mukesh, in my PC there’s an issue in loading Chrome, on single click is there any way to open from Selenium.
i’ll prefer to use ChromeBrowser.
Hi Girish,
If in your machine chrome is not properly installed then kindly reinstall it otherwise it will affect Selenium execution too.
working..
Cheers Benitto
hi Mukesh sir
explain how to write the code in POM multiple check box available in the webpage select one check box.
Hi Prabhakar, you can write for loop or while loop inside page which will select check box depends on the condition.
Hello Mukesh,
Please help me…
I am trying to execute my first selenium script..
1. I am downloaded JDK 12 Version.
2. I am using latest chromeversion 73
3. I am using Selenium- java 3.141.59
4. I am using Chrome Driver 73.0.3683.68
When I try to invoke the browser I am getting below error
Error: Unable to initialize main class practice
Caused by: java.lang.NoClassDefFoundError: org/openqa/selenium/WebDriver
Please help me o resolve the issue
Hi Raghava please use Java 8 for Selenium
Yes it is working fine with Java 8…Thank you so much
Great Raghava
Hi Mukesh, thank you for sharing this information.
I have doubt on setProperty statement in TestNG framework. Where should I place below line of code in testNG, is it Before or afrer @Test annotation?
System.setProperty(“webdriver.chrome.driver”, “path of the exe file\\chromedriver.exe”);
Please help me on this. I am getting error. Below is program.
package TestNG;
import org.testng.annotations.Test;
import org.testng.AssertJUnit;
import org.testng.Assert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
@Test
public class NewTestNG2 {
System.setProperty(“webdriver.chrome.driver”,”D:\\PracticeJava\\chromedriver.exe”); //getting error here
WebDriver driver = new ChromeDriver();
String AppUrl= “https://www.facebook.com/”;
public void f() {
driver.get(AppUrl);
driver.manage().window().maximize();
String ActTile=driver.getTitle();
System.out.println(ActTile);
String ExpTitle=”Facebook – log in or sign up”;
AssertJUnit.assertEquals(ActTile, ExpTitle);
WebElement username=driver.findElement(By.name(“email”));
WebElement pwd=driver.findElement(By.name(“pass”));
username.clear();
username.sendKeys(gmailID);
pwd.clear();
pwd.sendKeys(pwd);
driver.findElement(By.id(“loginbutton”)).click();
String FBTile=driver.getTitle();
driver.close();
}
}
Hi Anita, you can follow my framework code https://www.youtube.com/playlist?list=PL6flErFppaj0WwNOMFeXPVlNCDuJyPYFi
Im getting the below error….please help me
Exception in thread “main” java.lang.Error: Unresolved compilation problems:
WebDriver cannot be resolved to a type
ChromeDriver cannot be resolved to a type
Hi Leela,
Have you imported WebDriver and ChromeDriver libraries, if not kindly do it.
Hi Mukesh
I have a small doubt, i have done an automation for login page when it loads to firefox browser and launches the login page. In URL tab a robot logo is displaying, when i move cursor on it, it is showing message as Browser under remote control.
So what it means ?
Whether i’m using Selenium RC or it is just showing message?
Hi Qa,
It is informational message and it doesn’t affect your test automation. It is informing that browser is being handled without human intervention…:)
Hi Mukesh,
I am currently working on revamping the existing code using Chrome Driver(It was written for firefox earlier).Only recently I have started getting this error while loating elements, it was working fine till now:
org.openqa.selenium.WebDriverException: unknown error: Element … is not clickable at point (375, 58). Other element would receive the click: …
Can you please suggest me how to get this resolved? Do I need to use javascript executor for every element identification from now on?
Hi Prachi,
Kindly go through this link https://vistasadprojects.com/mukeshotwani-blogs-v2/how-to-solve-element-is-not-clickable-at-pointxy-in-selenium/
Hello
I am Getting below exception for this code while running chrometest
Exception in thread “main” java.lang.IllegalStateException: The driver executable does not exist: F:\Programs\Selenium Programs\Java\F:\Softwares Installed\chromedriver_win32\chromedriver.exe
at com.google.common.base.Preconditions.checkState(Preconditions.java:199)
at org.openqa.selenium.remote.service.DriverService.checkExecutable(DriverService.java:121)
at org.openqa.selenium.remote.service.DriverService.findExecutable(DriverService.java:116)
Hi Rishma,
Kindly check the path of chromedriver.exe
can anyone get me help using selenium with nodjs
Hi Saeed,
Please check this link http://webdriver.io/
Hi mukesh,
How to capture live http request and response using selenium.
Hi Sebastian,
You can try this http://www.seleniumeasy.com/selenium-tutorials/browsermob-proxy-selenium-example
Hi Mukesh,
I am getting the following exception.
org.openqa.selenium.SessionNotCreatedException: session not created exception
from tab crashed
(Session info: chrome=62.0.3202.62)
(Driver info: chromedriver=2.35.528161 (5b82f2d2aae0ca24b877009200ced9065a772e73),platform=Windows NT 6.1.7601 SP1 x86_64) (WARNING: The server did not provide any stacktrace information)
Command duration or timeout: 2.78 seconds
Build info: version: ‘2.35.0’, revision: ‘8df0c6bedf70ff9f22c647788f9fe9c8d22210e2’, time: ‘2013-08-17 12:46:41’
System info: os.name: ‘Windows 7’, os.arch: ‘amd64’, os.version: ‘6.1’, java.version: ‘1.8.0_161’
Driver info: org.openqa.selenium.chrome.ChromeDriver
at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:62)
at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:45)
at java.lang.reflect.Constructor.newInstance(Constructor.java:423)
at org.openqa.selenium.remote.ErrorHandler.createThrowable(ErrorHandler.java:191)
at org.openqa.selenium.remote.ErrorHandler.throwIfResponseFailed(ErrorHandler.java:145)
at org.openqa.selenium.remote.RemoteWebDriver.execute(RemoteWebDriver.java:554)
at org.openqa.selenium.remote.RemoteWebDriver.startSession(RemoteWebDriver.java:216)
at org.openqa.selenium.remote.RemoteWebDriver.(RemoteWebDriver.java:111)
at org.openqa.selenium.remote.RemoteWebDriver.(RemoteWebDriver.java:115)
at org.openqa.selenium.chrome.ChromeDriver.(ChromeDriver.java:161)
at org.openqa.selenium.chrome.ChromeDriver.(ChromeDriver.java:107)
at au.com.westpac.test.servlet.cases.GetJwtTest.main(GetJwtTest.java:16)
Hi Aparajia,
Please upgrade your chrome browser and try it once again.
Thank U for such a nice article.
Hi Vineet,
Thanks for your overwhelming comment…:)
I execute your given code, open window but not going to the website.occur this error. How to solve this problem. I need the code for open private(incognito) window in chrome
Starting ChromeDriver (v2.8.241075) on port 2352
Feb 01, 2018 6:15:33 PM org.openqa.selenium.remote.ProtocolHandshake createSession
INFO: Detected dialect: OSS
Exception in thread “main” org.openqa.selenium.WebDriverException: unknown error: Runtime.executionContextCreated has invalid ‘context’: {“auxData”:{“frameId”:”
Hi Sebastian,
First of all, chromedriver version which you’ve used is too old. Version 2.35 is latest one. Secondly, if you want to open chrome browser default in incognito mode then kindly use ChromeOptions with argument as –incognito
hi mukesh,
I will write this code but not open private window.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.remote.DesiredCapabilities;
public class Selenium
{
public static void main(String args[])
{
System.setProperty(“webdriver.chrome.driver”, “C:\\chrome driver.chromedriver.exe”);
WebDriver driver=new ChromeDriver();
DesiredCapabilities capabilities = DesiredCapabilities.chrome();
ChromeOptions options = new ChromeOptions();
options.addArguments(“incognito”);
capabilities.setCapability(ChromeOptions.CAPABILITY, options);
driver.get(“http://www.javatpoint.com”);
System.out.println(driver.getTitle());
}
}
Hi Sebastian,
You have to pass chromeoptions as argument to webdriver.
Hi mukesh,thank you
I get private window. I will given two url in open two tabs but two urls opened with single tab.how do you get two tabs in chrome .
driver.get(“http://google.com”);
System.out.println(driver.getTittle());
driver.findElement(By.cssSelector(“body”)).sendkeys(keys.CONTROL + “t”);
driver.get(“http://bing.com”);
System.out.println(driver.getTittle());
driver.findElement(By.cssSelector(“body”)).sendkeys(keys.CONTROL + “\t”);
System.out.println(driver.getTittle());
Hi Sebastian,
Use robot class to open another tab https://vistasadprojects.com/mukeshotwani-blogs-v2/robot-class-in-selenium-webdriver/ then switch driver control to new tab
Hi,
I tried running selenium with Chrome, however, getting the pop up as Google Chrome has stopped working. How to resolve this issue? Please help
Hi Prashant,
Kindly ensure that you are using latest Chrome and Chromedriver version in corresponding machine.
Hi mukesh,
I have one question but its not related to above topic.
actually i want to check weather my test case is pass or fail using listener in MS-test for selenium C#.
Hi Suraj
Apologies, I don’t support C#. But if it comes from Java then obviously TestNG help you.
Hi mukesh,
I have query on xpath’s
currently we are writing script with one xpath. if xpath is wrong or something changes in the application my script will fail. so i have scenario like i have to pass two xpaths where if one xpath fails find element method should take second xpath. is there any logic to do like this?
??
Hi Rakesh,
Use ‘or’ logic in while building xpath. Like //input[@value=’submit’ or @class=’submit_class’ or @title=’Submit’ or @type=’submit_button’]
Thanks for reply mukesh, you gave great idea but when we use following-siblings ,indexing or will not work know
Hi Rakesh,
You can give a try to text in your application also. You need to confirm with application ui developer that which all properties remain stable in ui. Because automation is carried out for regression testing and ui properties should be stable.
Hi Mukesh, in your “How to handle Element is no Clickable….” tutorial you haven’t used System.setProperty(); line. How to provide this particular functionality or have you done some pre-execution setting…????
Hi Shrikant,
In my blogpost, there are two things which you need to observe, Code snippet and video. I would suggest you to go through video first.
How to automate browser settings using selenium?
i have scenario like i want automate chrome browser settings->search proxy -> LAN btn-> Automatic Configuration->uncheck 1st checkbox-> select 2nd checkbox -> add address in Text box….? can we do it by using selenium?
Hi Akshay,
You can access Settings of chrome browser just like we do any web application.
Hi Mukesh, ur videos are very understandable and helps a new person to learn.
I am facing a issue I have recently installed selenium in my system and had run a basic script for which I am getting “Disable developer mode pop up is displayed due to which my case is getting failed.
Hi Rajesh,
Please go through this https://vistasadprojects.com/mukeshotwani-blogs-v2/disable-developer-mode-extension-in-chrome-selenium/
Hi
Nice article on Selenium, would like to know, how to handle captcha with selenium, any third party libraries are available for this?
Hi Satya,
Captcha is being added so that no automation and bot can get through authentication window/gateway. when you are testing any application, captchas have to be removed.
thank you mukesh i am able to solve the issue with the help of ur artical
Kudos…!!!
Exception in thread “main” java.lang.IllegalStateException: The path to the driver executable must be set by the webdriver.gecko.driver system property; for more information, see https://github.com/mozilla/geckodriver. The latest version can be downloaded from https://github.com/mozilla/geckodriver/releases
Hi Yasodhta,
Please provide gecko driver path. for more info, kindly check this link https://vistasadprojects.com/mukeshotwani-blogs-v2/use-firefox-selenium-using-geckodriver-selenium-3/
Hi Mukesh,
Its very good to see your blog and it is having a good concepts of automation with very clear explanation.
Please keep on posting new concepts.
Hi Haripriya,
Sure…:)
the way u explained really awesome.thanks mukesh
Hi Sekhar,
Thanks for your comments. You are always welcome to my bog…:)
Which chrome browser version is supported for selenium.
Hi Namani,
As such there is no specific details for same. Only thing is, always use latest version of chromedriver.
Very good information I got from this forum and thank you so much mukesh for all your patience in answering in everyone’s query.
Hi Pavithra,
Happy to read from you.
if i run the code in chrome than its showing plz enable the developer mode how to fix this issues
Hi Sonu,
Is it a pop up on right corner of screen ? If so then use chrome options as follows
ChromeOptions opts = new ChromeOptions();
opts.addArguments(“–disable-extensions”);
driver = new ChromeDriver(opts);
Hi Mukesh,
First-of-all,a big thanks on such a simple yet informative post.
Actually I am a beginner in Selenium/Automation, and I am trying to execute a simple script using Eclipse IDE on chrome browser to open up a page “google.co.in” as mentioned in the code below-:
import org.openqa.selenium.chrome.ChromeDriver;
public class TestChrome {
public static void main(String[] args) {
System.setProperty(“webdriver.chrome.driver”, “C:C:\\Users\\inpdhawan\\Downloads\\chromedriver_win32”);
// Initialize browser
ChromeDriver driver=new ChromeDriver();
}
But on Running the Script , even though Eclipse IDE shows no error but no webpage is poened up in the browser.
Can you please provide a solution to this.I am stuck here from past one week.
Regards
ZORO
Hi Zoro,
If I am seeing correctly then you have given an unappropriate path for chromedriver.exe. I can observe C drive in path mentioned twice. Moreover also add .exe at end of chromedriver_win32.
Hi Mukesh,
The workaround provided nailed the problem
🙂
Thanks a ton for your prompt reply
Cheers..!!!
Thank u mukesh,
What u said abt clearing history is correct but
I want to clear browser history. fllowing is the scenario
1.open browser
2.enter url (facebook)
3.navigate to gmail
4.clear history(facebook,gmail) which is currently opened by selenium web driver
how to automate this test script.I am used Actions class and key board events but i am not getting
Hi Sanjay,
Can you try with keyboard shortcut for clear history using robot class.
Hello Mukesh,
I want to clear chrome browser history.How to clear browser history
Hi Sanjay,
Selenium always opens new profile/fresh instance of browser by default along with no addons/extensions. So there is no need to clear history separately.
Hi forum,
public static void main(String[] args) {
WebDriver driver;
System.setProperty(“webdriver.chrome.driver”,”E://Selenium//Selenium Webdriver//chromedriver.exe”);
driver = new ChromeDriver();
driver.manage().window().maximize();
driver.get(“http://localhost:2424/login.do”);
Output-Browser is loaded but URL is not open
Error Message-Starting ChromeDriver (v2.9.248315) on port 20915
Exception in thread “main” org.openqa.selenium.remote.UnreachableBrowserException: Error communicating with the remote browser. It may have died.
Hi Nitin,
Which version of chromedriver and selenium are you using?
Its better if you giv a try to chromedriver.exe of 2.27 version and selenium 2.53.0
Hi Mukesh,
I’m getting error as
Starting ChromeDriver 2.24.417431 (9aea000394714d2fbb20850021f6204f2256b9cf) on port 14722
Only local connections are allowed.
Exception in thread “main” org.openqa.selenium.WebDriverException: unknown error: cannot find Chrome binary
Hi,
Kindly use chrome driver 2.25 version which will fix your issue.
Link for driver- Get 25 version only
https://chromedriver.storage.googleapis.com/index.html
Hi Mukesh,
I am getting following error
Exception in thread “main” org.openqa.selenium.SessionNotCreatedException: session not created exception
from unknown error: Runtime.executionContextCreated has invalid ‘context’: {“auxData”:{“frameId”:”9552.1″,”isDefault”:true},”id”:1,”name”:””,”origin”:”://”}
(Session info: chrome=54.0.2840.71)
(Driver info: chromedriver=2.21.371459 (36d3d07f660ff2bc1bf28a75d1cdabed0983e7c4),platform=Windows NT 6.1 SP1 x86_64) (WARNING: The server did not provide any stacktrace information)
Command duration or timeout: 1.48 seconds
Build info: version: ‘2.53.1’, revision: ‘a36b8b1’, time: ‘2016-06-30 17:32:46
Hi Sharath,
kindly use 2.25 version of chrome driver.
Hello,
I am not able to launch browser yet and getting error..(http://prntscr.com/chffda)
please help me , how to fix this ?
public class Sep13 {
public static void main(String[] args) {
System.setProperty(“webdriver.chrome.driver”,”C:\\Users\\naresh\\Downloads\\selenium-java-3.0.0-beta2\\chromedriver.exe”);
WebDriver driver= new ChromeDriver
driver.get(“http://www.google.com”);
driver.manage().window().maximize();
driver.getTitle();
Can you try with Selenium 2.53.1 http://selenium-release.storage.googleapis.com/index.html
It worked fine for me, thanks 🙂
Hi Mukesh,
Apart from this is there any method to invoke chrome browser?
Hi Rahul,
Another way is you can set chromedriver path in env variables and then everytinme it will start with driver.
Hi Mukesh,
Can you please tell from Selenium IDE how this will work to work on multiple browsers? Let’s say for example I test a web application/webpage and I want to run it in Chrome and IE, please explain step by step process with Python
Hi Dilshad,
For Java try below https://vistasadprojects.com/mukeshotwani-blogs-v2/cross-browser-testing-using-selenium-webdriver/
Apart from this is there any method to invoke chrome browser?
Hi Swetha,
You can set driver in path variable then you don’t have to mention path in test scripts.
I created a system variable with the name as ‘webdriver.chrome.driver’ and value as ‘D:\software\chromedriver64’
then in the system path variable i have added in the front %webdriver.chrome.driver%
Is this the right way ? Do We need to mention chroredriver.exe also somewhere
its not working the above way
Hi Gaurav if you keep driver path in System variable then it will work.
Hi,
I have provided the path for chromedriver in my code.
System.setProperty(“webdriver.chrome.driver”,”C:\\Users\\mitngh01\\Desktop\\Driver\\chromedriver.exe”);
System.out.println(“zxcv”);
WebDriver d = new ChromeDriver();
Still getting error as
Exception in thread “main” java.lang.NoClassDefFoundError: com/google/common/base/Function
at Selenium1.main(Selenium1.java:10)
Caused by: java.lang.ClassNotFoundException: com.google.common.base.Function
at java.net.URLClassLoader$1.run(Unknown Source)
at java.net.URLClassLoader$1.run(Unknown Source)
at java.security.AccessController.doPrivileged(Native Method)
at java.net.URLClassLoader.findClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
at sun.misc.Launcher$AppClassLoader.loadClass(Unknown Source)
at java.lang.ClassLoader.loadClass(Unknown Source)
… 1 more
kindly suggest.
Hi Mitali,
Selenium server is missing please add the jar and run again.
Apr 04, 2016 11:36:33 AM org.openqa.selenium.os.UnixProcess checkForError
SEVERE: org.apache.commons.exec.ExecuteException: Execution failed (Exit value: -559038737. Caused by java.io.IOException: Cannot run program “D:\sele_matter\chromedriver_win32.zip” (in directory “.”): CreateProcess error=193, %1 is not a valid Win32 application)
got this error???
is it fixed Sara?
Hi Mukesh,
I’m getting error as Cannot run program “D:\Selenium driver\chromedriver_mac32\chromedriver” (in directory “.”): CreateProcess error=193, %1 is not a valid Win32 application). could you please suggest any solution??
Hi Aarohi,
is this fixed?
Hi,
In Case of Maven Project, We are adding dependencies. So How to specify path for Chrome Driver?
Hi Manjunath,
In case of Chrome driver you can keep driver in resource folder then specify the path
Thanks Mukesh for your time and solution provided.
Your most welcome Gajendra 🙂
HI,
How would you make a test script to run on different versions of a web browser.
Say, i have a TC to be verified with chome 32 version only and same should work on ie 9, 10 and 11, or may be with little alterations.
i think i need to have different node machines with these versions of web browsers installed there.
Please clarify me on the best practice on this query.
Thanks in advance.
Gajendra
Hi Gajendra,
You can use https://saucelabs.com/ or https://www.browserstack.com/ which do the same thing.
If you want to implement the same in your local system then you should have different machines with different configurations.
Hope its clear for you 🙂
Thanks
Mukesh
Invalid port exiting …..
How to overcome this error
Hi Sagar,
If you using Java other than version 8 then uninstall the existing one -> Restart your system -> Install Java 8 and try the same once again.