How To Create Selenium Test using NUnit Framework
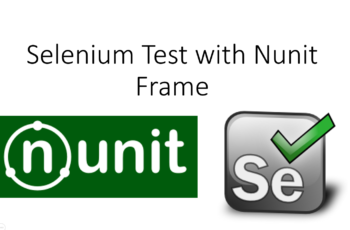
If you have worked on Java then you must have used JUnit or TestNG for unit testing. In the same way we have NUnit which works in slimier way. This post will guide you complete details about NUnit and how to build Selenium Test using NUnit Framework. Before we start let us discuss few points so that you can understand everything.
1-What is Unit Testing?
Unit Testing is a level of software testing where individual units or components of software are tested. In a Procedural Programming, a unit could be an entire module, but is more commonly an individual function or procedure. In Object-oriented Programming, a unit is often an entire interface, such as a class, but could be an individual method. Unit tests are short code fragments created by programmers or occasionally by White box testers during the development process. It forms basis for component testing
2-Types of unit test frameworks available.
A lot of unit testing frameworks are available for .NET. Most commonly used are
- MSTest
- NUnit
- NET or XUnit
3-Comparison between MSTest, NUnit and XUnit
4- What is NUnit
NUnit provides a console runner, which is used for batch execution of tests. The console runner works through the NUnit Test Engine, which provides it with the ability to load, explore and execute tests. Tests can run in parallel. Strong support for data driven tests. Supports multiple platform.
5-NUnit installation
Steps to install NUnit in visual studio.
Step 1: Create a C# project by using the steps mentioned in previous blog.
Step 2: Select the project created in right hand side and right click on it. Go to References -> Right click and select Manage NuGet Packages.
Alternative way is
- Click on Tool
- Select NuGet Package Manager.
- Select Manage NuGet Packages for solutions.
Step 3: In the next screen
- Click on Browse.
- Enter NUnit in search field and hit enter.
- Select the NUnit from the list of result.
- Click on Install.
Step 4: In next screen, click on OK.
Step 5: Once the NUnit is installed, we can see the following message.
Once NUnit is installed, let’s install NUnit Adapter. We can install NUnit Adapter in 2 ways
- Using Extension Manager.
- Using NuGet Package Manager.
Let’s see the installation of NUnit Adapter using Extension Manager.
Step 1:
- Click on Tools.
- Click on Extension and Updates.
Step 2: In the next screen,
- Click on Online
- Enter NUnit Adapter in Search field in the right side and hit enter.
- Once the result is found, Select the latest NUnit Test Adapter and click on It will start the download once u restart Visual Studio.
6-How to use NUnit with Selenium.
Let’s see how to use NUnit with selenium with an example.
Step 1: Create C# project by using steps mentioned in previous blog.
- Right click on Project.
- Select Add
- Select Class
Step 2: In the next screen,
- Select Visual C# items which will be selected by default.
- Select Class
- Enter the Name of the class in Name field
- Click on Add
Step 3: Write code which
- Opens a browser.
- Open some application URL and perform some action.
- Close the browser.
Let’s try this with Firefox browser.
Add Firefox driver in the similar way we added chrome driver in previous blog.
Code without Annotations look like.
IWebDriver driver; //Declare WebDriver instance public void Initialize() { //create a firefox driver instance driver = new FirefoxDriver(); } public void LaunchApp() { //Maximize the browser driver.Manage().Window.Maximize(); //Launch a website driver.Url = "https://vistasadprojects.com/mukeshotwani-blogs-v2/"; } public void ShutDown() { driver.Close(); }
Code with Annotations looks like
Step 4: Build the class.
- Click on Build
- Select Build Solution.
Once Build is completed, we may get CS5001 error which says Main method is missing.
In order to resolve it
- Go to Project.
- Select NUnit_Project Properties.
In the next screen,
Select Class Library and save the changes (Ctrl +S).
Step 5: Once build is completed, open Test Explorer.
- Click on Test
- Select Windows
- Click on Test Explorer.
Test Explorer will be opened in left side which contains all the tests present
Step 6: Select the test which u want to run and click on Run Selected Test.
‘
Test will start its execution.
Once execution is completed we can see the result as below.
Hi,
Generally, can we create a maven project using selenium with C#.
Thanks
Hi Prasad,
There are some theories of Maven support for C# but I don’t think of any fully practical solution for C#. If you find something solid then let me know…:)