Browser Navigation commands in Selenium with C#
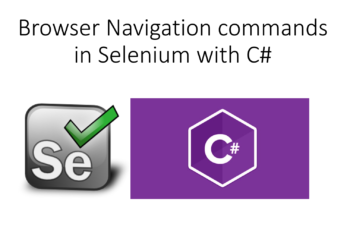
Selenium WebDriver has already provided many browser Navigation commands like Back, Forward, Refresh method which help us to navigate easily. This post will walk you through different type of Browser Navigation commands in Selenium with C#.
Browser Navigation commands in Selenium with C#
Navigation Commands.
Browser Navigation commands are used to perform navigation operations in browser. Navigation Commands in C# are implemented using INavigation Interface. Navigation commands available in Selenium with C# are
1 GoToUrl.
2 Back.
3 Forward
4 Refresh.
GoToUrl – This command is used to navigate to particular URL or website.
Signature is void INavigation.GoToUrl(String URL) – This command takes URL as string input parameter.
Syntax: driver.Navigate().GoToUrl(URL);
where URL is application url.
Back – This command is used to navigate back to previous webpage in browser history. This command is very similar to Back button in browser.
Signature is void INavigation.Back() – This command will not accept any parameter.
Syntax: driver.Navigate().Back();
Forward -This command is used to move or go to next webpage present in browser history. This command is very similar to Forward button in browser.
Signature is void INavigation.Forward() – This command will not accept any parameter.
Syntax: driver.Navigate().Forward();
Refresh – This command is used to refresh the webpage.
Signature is void INavigation.Refresh() – This command will not accept any parameter.
Syntax: driver.Navigate().Refresh();
Usage of Navigation Commands with example
Step 1: Create a C# project using steps discussed before. Add Selenium and Internet Explorer driver.
Step 2: Write a program which uses 4 Navigation commands.
Step 3: Code explanation is
Note- In this example I will take IE browser example and I have also detailed post I have discussed different challenges which I faced while working with IE browser and this is also the most frequently asked questions in Interview.
//declare a driver instance IWebDriver driver; //create IE driver instance driver = new InternetExplorerDriver(); //Open learn-automation.com driver.Navigate().GoToUrl("https://vistasadprojects.com/mukeshotwani-blogs-v2/"); //find Element with xpath and click on it driver.FindElement(By.XPath("//*[text()='Selenium Videos']/..")).Click(); //click on back button using navigate driver.Navigate().Back(); //click on forward button using navigate driver.Navigate().Forward(); //click on refresh driver.Navigate().Refresh(); //close the driver instance driver.Close();
Step 4: Build the code.
- Click on Build.
- Select Build Solution.
Note: If u get any “System.InvalidOperationException” then
- Click on Tools.
- Select Internet Options.
Then
- Click on
- Select each Internet, Local Intranet, Trusted sites, Restricted Sites.
- Set same Security level for all 4. Enable /Disable Protected Mode for all 4.
- Click on
- Click on OK.
Once settings are changed, Build again.
Once the build is successful. Start the execution by clicking on Start button.
Output:
- IE browser will be launched.
- Learn-automation.com is opened.
- It will click on SELENIUM VIDEOS link.
- Browser will go back to Home page.
- Once the page is loaded then again browser will open Selenium videos page.
- Once Selenium Video Page is loaded, webpage will be refreshed.
- Browser will be closed.
Hi Mukesh,
I have a small doubt, for one of my testing I need test the privacy Policy, where If I click a link in my webpage, It opens a new tab with privacy policy. Can you please help me how to test that functionality
Hi Mythri,
This scenario is of window handling where you need to switch driver to new window/tab and verify things on corresponding page. Then close this window and switch back to previous one.
Awsume explained
Hi Sharrif,
Very very thanks for your appreciation. You are always welcome to my blog…:)