Page Object Model using Selenium Webdriver and Implementation
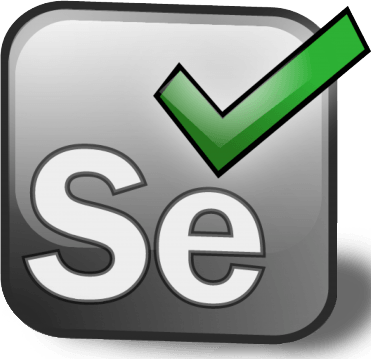
In this post, we are going to discuss a very important topic or concept called Page Object Model, which you have to use in your project now or in the future if you are trying to create a framework.
Many companies use Page Object Model is a design pattern to store all locators and methods in separate Java class and we can use the same class in different test cases.
I get so many questions regarding Page Object Model framework but let me make it clear that Page Object model is just a design pattern, not a framework. There are multiple design pattern available for Java-like Singleton design pattern, Structural design pattern and so on. You can read about different design patterns in Java from this link.
We will also use Page Object Model in Selenium to make our framework efficient and robust. I also have detailed video series on Hybrid Framework in Selenium on my youtube channel where we have used the same pattern. P
This is one the most important questions in interviews as well because now a day every company is trying to switch from traditional object repository to POM.
What is Page Object model using Selenium Webdriver?
1- It is a design pattern, which will help you to maintain the code and avoid code duplication, which is a crucial thing in Test automation.
2- You can store all locators and respective methods in a separate class and call them within the test in. The benefit from this will be, if any changes in web element locators then you do not have to modify the test simply modify the respective page and that all.
For example, earlier id was username and if id changed to uname then you do not have to change all the tests. You can use change the id in the respective page.
3- You can create a layer between your test script and application page, which you have to automate.
4- In other words, it will behave as Object repository where all locators are saved.
“Please don’t get confused if you are using Object repository concept which is property file, then do not use the Page Object model because both will serve the same purpose. You can use the file to store other configuration ”
Implementation of Page Object Model using Selenium Webdriver
If you want to implement the Page Object model then you have two choices and you can use any of it.
1 – Page Object model without PageFactory
2- Page Object Model with Pagefactory.
Personally, I am a big fan of Page Factory, which comes with Cache Lookup feature, which allows us to store frequently used locators in the cache so that performance will be faster. We will discuss both of them in this post and you can implement any of them based on your preferences.
Page Object Model using Selenium Webdriver without PageFactory.
Let’s take a very basic scenario so that you can relate to any application. Consider you have login page where username, password, and login button is present.
I will create a separate Login Page and will store three locators, and we will create methods to access them. Kindly refer below screenshot for reference.
Now I want to design a test case so that I can use the Login class, which I created and can call the methods accordingly.
This Login class can be used by all the test scripts, which you will create in future so if any changes happened in DOM then you have to update only Login Class not all the test cases.
I have created Youtube Video for the same
Program for Page Object Model using Selenium Webdriver without Page factory
package com.wordpress.Pages; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.support.CacheLookup; import org.openqa.selenium.support.FindBy; import org.openqa.selenium.support.How; /** * @author Mukesh_50 * * * This class will store all the locator and methods of login page * */ public class LoginPage { WebDriver driver; By username=By.id("user_login"); By password=By.xpath(".//*[@id='user_pass']"); By loginButton=By.name("wp-submit"); public LoginPage(WebDriver driver) { this.driver=driver; } public void loginToWordpress(String userid,String pass) { driver.findElement(username).sendKeys(userid); driver.findElement(password).sendKeys(pass); driver.findElement(loginButton).click(); } public void typeUserName(String uid) { driver.findElement(username).sendKeys(uid); } public void typePassword(String pass) { driver.findElement(password).sendKeys(pass); } public void clickOnLoginButton() { driver.findElement(loginButton).click(); } }
TestCase using Page Object Model using Selenium Webdriver
/** * */ package com.wordpress.Testcases; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.testng.annotations.Test; import com.wordpress.Pages.LoginPage; /** * @author Mukesh_50 * */ public class VerifyWordpressLogin { @Test public void verifyValidLogin() { WebDriver driver=new FirefoxDriver(); driver.manage().window().maximize(); driver.get("http://demosite.center/wordpress/wp-login.php"); LoginPage login=new LoginPage(driver); login.clickOnLoginButton(); driver.quit(); } }
Page Object Model using Selenium Webdriver with Page Factory
Selenium has built in class called PageFactory, which mainly serve Page Object model purpose, which allows you to store elements in cache lookup.
The only difference, which you will get without PageFactory and with PageFactory, is just initElement statement.Let us check the code and will see what changes required for with PageFactory Approach.
Another YouTube video for Page Object model with Page Factory
Code for Page Object Model Using Selenium Webdriver using Page Factory
package com.wordpress.Pages; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.support.CacheLookup; import org.openqa.selenium.support.FindBy; import org.openqa.selenium.support.How; public class LoginPageNew { WebDriver driver; public LoginPageNew(WebDriver ldriver) { this.driver=ldriver; } @FindBy(id="user_login") @CacheLookup WebElement username; @FindBy(how=How.ID,using="user_pass") @CacheLookup WebElement password; @FindBy(how=How.XPATH,using=".//*[@id='wp-submit']") @CacheLookup WebElement submit_button; @FindBy(how=How.LINK_TEXT,using="Lost your password?") @CacheLookup WebElement forget_password_link; public void login_wordpress(String uid,String pass) { username.sendKeys(uid); password.sendKeys(pass); submit_button.click(); } } Test Case using Page Factory package com.wordpress.Testcases; import org.openqa.selenium.WebDriver; import org.openqa.selenium.support.PageFactory; import org.testng.annotations.Test; import com.wordpress.Pages.LoginPage; import com.wordpress.Pages.LoginPageNew; import Helper.BrowserFactory; public class VerifyValidLogin { @Test public void checkValidUser() { // This will launch browser and specific url WebDriver driver=BrowserFactory.startBrowser("firefox", "http://demosite.center/wordpress/wp-login.php"); // Created Page Object using Page Factory LoginPageNew login_page=PageFactory.initElements(driver, LoginPageNew.class); // Call the method login_page.login_wordpress("admin", "demo123"); } }
I hope you have enjoyed the article and if yes then feel free to share this article with other and let me know your feedback in below comment section.
Nice one)))
Hi Mukesh,
Hope you are doing good…
I have followed the same steps to create the POM. I am getting illegalStateException error even though used the chromedriver path correctly. I am using the chrome browser instead of firefox. please assist me where I am doing wrong.
Chrome exe v88
chrome v 88
Selenium jar 3.41.59
TestNG v7.3
Hi Manju,
Check whether path of the driver is correct and it includes file extension too. Let me know with your observations.
Please help me understand how driver instance is passed as a constructor.I am a new bee to Java and Selenium.
I understand that constructor is used to initialize variable to a value, we can achieve this using getters and setters.
But unable to relate it(constructor) with WebDriver driver.
Where in I have a different class of driver.
Please help me with some diagram.
Hi Shubhangi,
Please go through this link https://www.youtube.com/watch?v=EZJiW5oM2Ks&list=PL6flErFppaj0WwNOMFeXPVlNCDuJyPYFi&index=3 Here I’ve explained the creation of page object class where I used driver object passed to the constructor.
Hi mukesh ,
A small clarification,
In the POM eg without using page factory
U have not called the logintowordpress(string username,string password) method from verifywordpresslogin class . Can u pls verify .
Regards
Varsha Krishna Kumar
Hi Varsha,
Thanks for bringing up this. I’ll update it…:) Please feel free to ask your doubts and queries
Hi Mukesh,
How do i resolve this issue ….Element not clickable at point (1059, 232). Other element would receive the click
Hi Sonali,
Please check this link https://vistasadprojects.com/mukeshotwani-blogs-v2/how-to-solve-element-is-not-clickable-at-pointxy-in-selenium/
How can we apply waits in page object classes? I am applying webdriverwait and getting null pointer exception. can you take one example of clicking button and then waiting for any link to visible. I can use wait in normal framework but cannot in page object model.
Hi Gaurav,
I’ll post more contents related to framework soon…:)
HI Mukesh
Please consider now we have many class for page object.
Ex: The second page is depends on first page and the third page is depends on second page,
In this case we want to pass driver instance for all the page object class.
should we create object for three different class or any other way is there .
Please explain other solution without creating object for second class.
How to get webdriver instance form page1.?
Hi Mahesh,
Page object model, usually driver needs to be passed as a parameter
Nice Sir, Very simple explaination that we required. Thnaks
Hi Ankit,
You’re always welcome to ask your doubts in automation…:)
Which is better to implement Page object model using repository or using Page Factory?
Hi Harshit,
It’s better to use Page Factory
Hi Mukesh,demo site is not working its giving below error
demosite.center is currently unable to handle this request. HTTP ERROR 500.
How to resolve this?
Hi Soni,
For time being, you can use this site https://opensource-demo.orangehrmlive.com/ for practice purpose.
Yaa nice dude , its really help us …thanks for your dedications towards scripts
Thanks Himansu…:)
How do I automate OTP login via selenium, I want to automate Login via OTP in a website
Hi Suyash, avoid automating OTP scenarios.
Hi Suyash/ Mukesh
The below site will be helpful for authenticating OTP
https://aerogear.org/docs/guides/AeroGear-OTP/
I have tried and it works
Hi Mali,
I really appreciate your efforts for helping others…:)
Hi mukesh,
Tq,Very helpfull
Hi Uk,
You are most welcome…:)
What does this error define
[TestNG] Running:
C:\Users\pavithra\AppData\Local\Temp\testng-eclipse-594331317\testng-customsuite.xml
FAILED: verifyValidLogin
java.lang.IllegalStateException: The path to the driver executable must be set by the webdriver.gecko.driver system property; for more information, see https://github.com/mozilla/geckodriver. The latest version can be downloaded from https://github.com/mozilla/geckodriver/releases
Hi Pavithra,
This is line java.lang.IllegalStateException: The path to the driver executable must be set by the webdriver.gecko.driver system property; which tells path of geckodriver path is not correct. Please refer this link too https://vistasadprojects.com/mukeshotwani-blogs-v2/use-firefox-selenium-using-geckodriver-selenium-3/
Kindly put geckodrive.exe file into your user folder then try it again(sometimes non admin user doesn’t gets proper privileges into Program Files folders) . Also check syntax and location of driver file is correct.
Above as u replied me yesterday -I have save the gecko driver in User not in c driver
Hi Pavithra,
I don’t see any other error / exceptions apart from gecko driver file path issue. Because exception message itself mentions file path issue.
Hi, I have tried the same as above but its shows error
FAILED: verifyValidLogin
java.lang.IllegalStateException: The path to the driver executable must be set by the webdriver.gecko.driver system property; for more information, see https://github.com/mozilla/geckodriver. The latest version can be downloaded from https://github.com/mozilla/geckodriver/releases
Hi Pavithra,
Issue clearly corresponds to wrong gecko driver path (The path to the driver executable must be set by the webdriver.gecko.driver system property).
Thanks Mukesh , Very helpful vedio.
Significant contribution to Testing Community.
Thanks..:)
Hi Mukesh, my script has been implemented using data from excel, i too want refactor my code using POM, but facing issues, Is there any tutorial which can help me for this?,
Else the above explanation is good enough for me when im not fetching my data from excel.
Thanks,please do help me
Hi Neelima,
As per my understanding, you want to change your existing framework to Page Object Model, right?
Hi ,
Nice tutorial. Was searching for something so clear since long 🙂
Hi Shivani,
I am happy to see that my blog post has helped you. Many many thanks for your appreciating comments.
awesome!!! video with no parameters
Thanks JK 🙂
Hi Mukesh,
If i need to pass data from excel sheet for the above framework.
How do i go about it.
Hi Pooja,
Please refer this link https://vistasadprojects.com/mukeshotwani-blogs-v2/read-and-write-excel-files-in-selenium/
Hi Mukesh, Nice tutorial.Is there a way to check if entire page has been loaded including all frames in page without using webdriverwait or implicit wait. If yes how.
Hi Daisy,
You can use javascript call for document.readyState to be equal to complete. But anyway implicit wait internally uses same.
Hi Mukesh,
Your comment is awaiting moderation.
if i execute my selenium test whenever i found even one bug entire automation test had stopped . next test case does not get executed.
so please tell after finding bug developer should fix the bug then only we excute test script or we just capture that bug and execute next script.
if you try to say just capture the bug and eexcute next line script how we caputre the bug and execute next script
Hi Nisha,
In this case, you can put exception catch after every action. If you catches any exception which usually happens when bug comes out(as in your case) then after this next step of execution will proceed. You need to design your framework in a way so that each action goes through exception check.
Hi mukesh ji, how can we automate homepage by using pagefactory, for ex:i need to count the no of socialicons in a given homepage?
Hi Kanchana,
First understand the fact that there is no relation between pagefactory and count of no. of socialicons. You can check no href properties or anchor links(depends on your application page) after navigating to corresponding controls. To get how to verify those links, please visit here https://vistasadprojects.com/mukeshotwani-blogs-v2/find-broken-links-using-selenium/
Hi Mukesh…Working on ur assignment .was just wondering how do i write text in paragraph…it does not work with send keys:(
Hi Anusha,
Are you using any web based editor tool like CK Editor? If not then please explain scenario.
You are the best??????sooper sooper sooper??????
Hi Anusha,
Thanks Anusha…your positive comments are driving me forward.
Hi, your article is really helpful.
I need to read the object from excel file.
@FindBy(how=How.XPATH,using=”.//*[@id=’wp-submit’]”)
here, I need to get “.//*[@id=’wp-submit’]” from excel file with page factory concept. Could you please suggest some ways to do it? I tried it. its throwing some error.
Hi John,
Please go through second section on this link https://vistasadprojects.com/mukeshotwani-blogs-v2/read-and-write-excel-files-in-selenium/
For an example, you can use it this way —>>> By Add_Button = By.xpath(object property from Excel);
Add_Button is locator. Now you can use it as driver.findElement(AddButton).click();
THis is nice explanation but would be good if you can explain the with factory code. The first program is very much clear.
But the POM with object factory has many new syntaxes. if you add a paragraph for that it would be good. Which one is more used in industry ?
We use Page Object model with Page Factory it also helps in performance.
public void login_wordpress(String uid,String pass)
{
username.sendKeys(uid);
password.sendKeys(pass);
submit_button.click();
}
Shouldn’t the above function be like this
public void login_wordpress(String uid,String pass)
{
driver.findElement(username).sendKeys(uid);
driver.findElement(password).sendKeys(pass);
driver.findElement(submit_button).click();
}
Yes Skay I have to modified the code.. Thanks for update.
Here it seems for every test case we are initializing and creating new object for driver. How to we maintain driver object as common for all pages and tests
Hey Praneeth,
You can check below video for this.
Dear Mukesh Otwani,
iam creating “LoginPageNew ” like same as u ,
and calling directly methods in another class.
its showing null point Exception (but i am not create any “Page Factory” class)
” Ur class are very good ,iam following that ,
i am not understand “Page Factory” class
Hi Sravan,
Make sure webdriver is passed in everypages then you wont find any null pointer exception.
VERY WELL EXPLAINED. ALL SCRIPTS RAN WELL.
THANK YOU SO MUCH
HI Nidhi,
Good to hear that. CHeers.