How to run Selenium Webdriver test in IE browser / Internet Explorer Driver
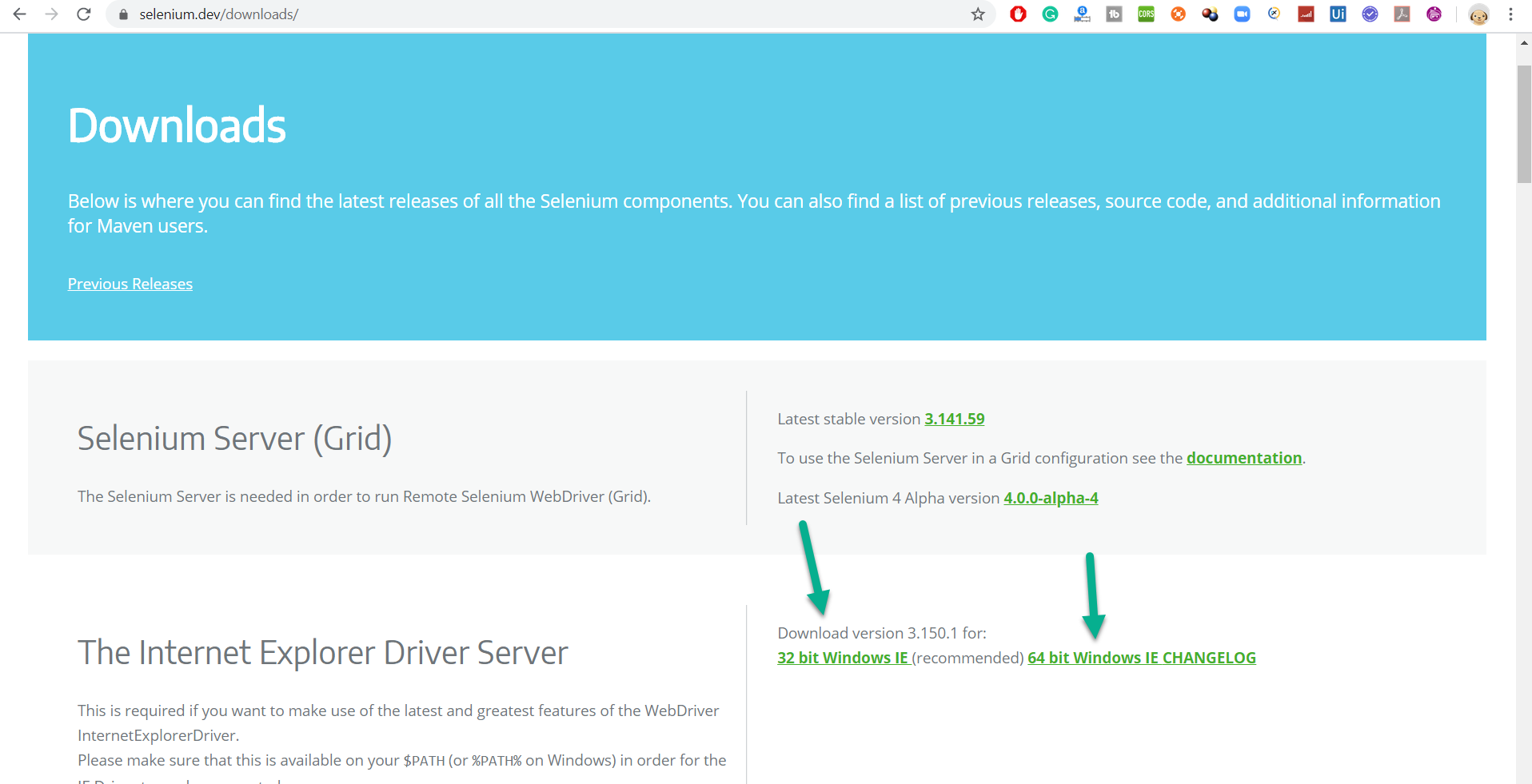
This post will guide you How to Launch IE Browser in Selenium Webdriver and recently Selenium started supporting the Microsoft Edge browser as well which is an added advantage for Windows 10 users.
In the previous post, we have already discussed for Chrome, Firefox, Edge Browser with third-party drivers. We will follow the same process for IE Browser as well.
You will also face few issues with IE Browser which I already listed in the below post so if you face any issue then do check out below link.
Challenges you will face while working with IE browser
How To Launch IE Browser in Selenium
Step 1-Download IE driver for Selenium
Open-http://docs.seleniumhq.org/download/
Depends on your system requirement download the respective driver for Windows 32 download driver 32-bit driver same applies for 64 as well
A download window will open, wait till the download complete. Once you get zip file unzip the same you will get IEDriverServer.exe
Step 2- Write script for IE browser in Selenium
As we have seen in the last post for chrome, we talked about exception also same applies for IE also.
We need to set variable webdriver.ie.driver
Program for running IE Browser using Selenium
package demotc;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.ie.InternetExplorerDriver;
import org.testng.annotations.Test;
public class IEBrowser {
@Test
public void test12() throws Exception{
// Initialise browser
WebDriver driver=new InternetExplorerDriver();
// Load google.com
driver.get("http://www.google.com");
// close browser
driver.close();
}
}
Step 3- Run Program using Java application-
Right-click on program> run as >Java application
Output:
Your program will fail because we haven’t set IE driver path so let’s set the path using setProperty method
package demotc;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.ie.InternetExplorerDriver;
import org.testng.annotations.Test;
public class IEBrowser {
@Test
public void test12() throws Exception{
// set driver path
System.setProperty("webdriver.ie.driver","driver path\\IEDriverServer.exe");
// Initialise browser
WebDriver driver=new InternetExplorerDriver();
// Load google.com
driver.get("http://www.google.com");
// close browser
driver.close();
}
}
Now your program will run successfully. 🙂
Some key points while working with IE Browser in Selenium.
- IE browser is slow as compared to other browsers.
- Your browser zooming level should be set to 100 % otherwise, you will get an exception.
- You have to check your security setting also in IE. While running IE browser in Selenium your all zones should be either enabled or disabled. If not then again you will get an exception and your test cases will fail.
Hi Mukesh,
Few locators(xpath) are working fine in Chrome and Firefox but failing in IE browser during execution.. is there any way to implement it to be stable for all browsers or else its better to move for cssselector approach?
Hi Sathish,
In order to cop up with IE along with other browsers, i would recommend you to use CSSSelector
Thanks you so much Mukesh….
Thank you so much Mukesh Otwani for providing a valid information
Hi Bangarraju,
You are always welcome…:)
Hi Mukesh,
I have entered the code as mentioned below
public static void main (String args[])
{
System.setProperty(“webdriver.ie.driver”,”C:\\SeleniumDrivers\\IEDriverServer.exe”);
WebDriver driver = new InternetExplorerDriver();
driver.get(“http://www.google.co.in”);
}
After executing, i am displayed with a new internet explorer browser with the message “This is the initial start page for the WebDriver server”. Waited for 10 mins, still it is not redirected the user to “google home page”.
Please help me in the same
Hi babu,
Kindly check browser zoom settings along with Protected Mode settings. for details, please visit this link https://vistasadprojects.com/mukeshotwani-blogs-v2/challenges-with-ie-browser-in-selenium-webdriver/
All your articles very useful and knowledgeable.
Doing really a great job.
Thanks Mate
Hi,
Started with selenium recently, and found your website. It is really fabulous, and you really are doing a great job sharing your knowledge beautifully.
Hi Su,
Thanks for your comments…
Hi,
I am getting below error. Please help me.
Started InternetExplorerDriver server (64-bit)
2.53.1.0
Listening on port 26458
Only local connections are allowed
Exception in thread “main” org.openqa.selenium.remote.SessionNotFoundException: Unexpected error launching Internet Explorer. Protected Mode settings are not the same for all zones. Enable Protected Mode must be set to the same value (enabled or disabled) for all zones. (WARNING: The server did not provide any stacktrace information)
Command duration or timeout: 1.47 seconds
Hi Madhur,
Please follow below post
https://vistasadprojects.com/mukeshotwani-blogs-v2/challenges-with-ie-browser-in-selenium-webdriver/
is it not possible to set zooming level 100% and security settings in ie using selenium?
Yes you can do that using below code
DesiredCapabilities caps = DesiredCapabilities.internetExplorer();
caps.setCapability(“EnableNativeEvents”, false);
caps.setCapability(“ignoreZoomSetting”, true);
// Initialize InternetExplorerDriver Instance using new capability.
WebDriver driver = new InternetExplorerDriver(caps);
Hi Mukesh,
I was successfully able to run IE browser and navigate to a page. But my browser is not getting closed even after using driver.close() method. However it was working fine in chrome. please let me know the reason.
Hi Ajay,
quit will work in this case.
Hi mukesh,
please go through the below code when i run this i am getting the title as Webdriver in the console if i run same code using other browsers i am getting the correct output.please let me know what is wrong with the code
{
System.setProperty(“webdriver.ie.driver”, “F:\\selenium drivers\\IEDriverServer.exe”);
WebDriver driver=new InternetExplorerDriver();
driver.get(“https://vistasadprojects.com/mukeshotwani-blogs-v2”);
System.out.println(“Title is ” +driver.getTitle());
}
}
Hi Shobha,
Make below changes in IE and then run the same code again http://learn-automation.usefedora.com/courses/selenium-frameworks-and-selenium-question-answers
I m having below code, but facing the below issue. I have latest ie driver files, latest selenium external jar files. Please help me to understand.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.ie.InternetExplorerDriver;
public class ie
{
public static void main(String args[])
{
System.setProperty(“Webdriver.ie.driver”, “D:\\IEDriverServer.exe”);
WebDriver driver = new InternetExplorerDriver();
driver.manage().window().maximize();
driver.get(“https://paytm.com/”);
}
}
Issue faced:
Exception in thread “main” java.lang.IllegalStateException: The path to the driver executable must be set by the webdriver.ie.driver system property; for more information, see https://github.com/SeleniumHQ/selenium/wiki/InternetExplorerDriver. The latest version can be downloaded from http://selenium-release.storage.googleapis.com/index.html
at com.google.common.base.Preconditions.checkState(Preconditions.java:199)
at org.openqa.selenium.remote.service.DriverService.findExecutable(DriverService.java:109)
at org.openqa.selenium.ie.InternetExplorerDriverService.access$0(InternetExplorerDriverService.java:1)
at org.openqa.selenium.ie.InternetExplorerDriverService$Builder.findDefaultExecutable(InternetExplorerDriverService.java:167)
at org.openqa.selenium.remote.service.DriverService$Builder.build(DriverService.java:296)
at org.openqa.selenium.ie.InternetExplorerDriver.setupService(InternetExplorerDriver.java:251)
at org.openqa.selenium.ie.InternetExplorerDriver.(InternetExplorerDriver.java:172)
at org.openqa.selenium.ie.InternetExplorerDriver.(InternetExplorerDriver.java:146)
at paytm.ie.main(ie.java:12)
Hi,
PLease make below change
Old code
System.setProperty(“Webdriver.ie.driver”, “D:\\IEDriverServer.exe”);
New code
System.setProperty(“webdriver.ie.driver”, “D:\\IEDriverServer.exe”);