How to capture screenshot for failed test cases in Selenium Webdriver
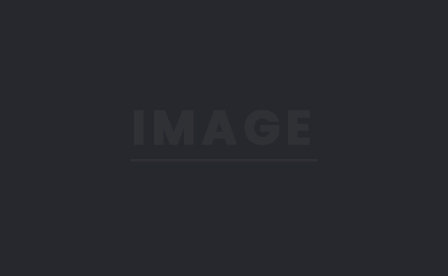
Writing Selenium Webdriver script is not enough everyone can design a script nowadays. We need to design the script in such a way that we can utilize script code as much as possible. This article will talk about the Capture screenshot in selenium for failed test cases.
I am a big fan of screenshots in Automation because it helps me a lot to identify the exact issue.
Generally, scripts fail in 2 situations.
1-If script has some issue (some locator has been changed or application has some changes)- In this case, we need to maintain our Selenium script.
2-Due to application issue- In this case, we need to inform to respective point of contact (Manual Tester or Developer)
How to Capture screenshot in selenium for failed test cases
Previously I have covered a post on capture screenshot in Selenium so if you have not gone through the previos post then I will highly recommend you to please go through the post and youtube as well.
https://vistasadprojects.com/mukeshotwani-blogs-v2/how-to-capture-screenshot-in-selenium-webdriver/
Today we will see something different How to capture a screenshot for failed test cases in Selenium Webdriver.
Here I will be using two new topics which will help us to achieve the same.
1-We will use ITestResult Interface which will provide us the test case execution status and test case name.
Please refer official doc for ITestResult
2- @AfterMethod is another annotation of TestNG that will execute after every test execution whether test case pass or fail @AfterMethod will always execute.
Please refer official doc for @AfterMethod
If you are new to TestNG and want to explore more on TestNG then I will recommend you to please go through the below TestNG topics.
I have covered a couple of topics for TestNG. You can check all TestNG Tutorial here
Now let’s get started and will see through a complete program.
If you want to refer to video for the same then refer to the YouTube video
Program to Capture screenshot in selenium for failed test cases
// Create a package in eclipse package captureScreenshot; // Import all classes and interface import java.io.File; import library.Utility; import org.openqa.selenium.io.FileHandler; import org.openqa.selenium.By; import org.openqa.selenium.OutputType; import org.openqa.selenium.TakesScreenshot; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.testng.ITestResult; import org.testng.annotations.AfterMethod; import org.testng.annotations.Test; public class FacebookScreenshot { // Create Webdriver reference WebDriver driver; @Test public void captureScreenshot() throws Exception { // Initiate Firefox browser driver=new FirefoxDriver(); // Maximize the browser driver.manage().window().maximize(); // Pass application url driver.get("http://www.facebook.com"); // Here we are forcefully passing wrong id so that it will fail our testcase driver.findElement(By.xpath(".//*[@id='emailasdasdas']")).sendKeys("Learn Automation"); } // It will execute after every test execution @AfterMethod public void tearDown(ITestResult result) { // Here will compare if test is failing then only it will enter into if condition if(ITestResult.FAILURE==result.getStatus()) { try { // Create refernce of TakesScreenshot TakesScreenshot ts=(TakesScreenshot)driver; // Call method to capture screenshot File source=ts.getScreenshotAs(OutputType.FILE); // Copy method specific location here it will save all screenshot in our project home directory and // result.getName() will return name of test case so that screenshot name will be same try{ FileHandler.copy(source, new File("./Screenshots/"+result.getName()+".png")); System.out.println("Screenshot taken"); } } catch (Exception e) { System.out.println("Exception while taking screenshot "+e.getMessage()); } } // close application driver.quit(); } }
The above code will execute fine and if the test case will fail it will capture the screenshot. Check below screenshot
The above code is fine but still, we need to enhance our code so that we can reuse it.
We will create a Utility class that will have one method which will capture the screenshot.
Code to create a method for screenshot
package library; import java.io.File;import org.apache.commons.io.FileUtils; import org.openqa.selenium.OutputType; import org.openqa.selenium.TakesScreenshot; import org.openqa.selenium.io.FileHandler; import org.openqa.selenium.WebDriver; public class Utility { public static void captureScreenshot(WebDriver driver,String screenshotName) { try { TakesScreenshot ts=(TakesScreenshot)driver; File source=ts.getScreenshotAs(OutputType.FILE); FileHandler.copy(source, new File("./Screenshots/"+screenshotName+".png")); System.out.println("Screenshot taken"); } catch (Exception e) { System.out.println("Exception while taking screenshot "+e.getMessage()); } } }
You call the above method in the below format
Utility.captureScreenshot(driver,"name of screenshot");
Hope the above article will help you in the Interview or in your automation test framework.
Comment below if any queries or suggestions or Feedback.
For More updates Learn Automation page
For any query join Selenium group- Selenium Group
org.openqa.selenium.TakesScreenshot.getScreenshotAs(org.openqa.selenium.OutputType)” because “ts” is null
getting this error after run the testcase.
Hi Hitesh, please share the code to email mukeshotwani@learn-automation.com
Null pointer exception is very common java exception which is because variable have null value.
Hi Mukesh,
Actually that issue has resolved now but its i am running multiple users via excel and the testcases are depends on that users. So the issue is i am getting only single screenshot of failed testcase which is running in the last.
Hi Hitesh,
Please share your code because scenario which you have mentioned here is understandable but there is no information about how your framework configured. You need to check your framework thoroughly.
can you share, how you resolved your issue ?
Hi Ramandeep,
If you are also facing the same issue then you need to have screenshots name dynamically like adding some timestamp in filename.
could you please tell how this issue is resolved
Hi Hitesh, please explain your query in detail.
Hi ,
How to trigger the HTML test execution report automatically to or mailbox using maven and testng
Hi Mahesh,
You can refer below links
https://vistasadprojects.com/mukeshotwani-blogs-v2/send-report-through-email-in-selenium-webdriver/
Hi Mukesh Greetings & my best wishes.
Can we do the same in C# as well?
If yes, could you please do a video or write a blog on the same.
Hi Shiva,
You can use OnException method from EventFiringWebDriver class where you can inject screenshot taking mechanism. Link is here
I’ll plan one video on this topic too.
Hi Mukesh,
Is there any prerequisite for this screenshot code, saying so, Do we need any specific version of Apache POI of testng for this code?
Hi Rahul,
Apache POI is to deal with Microsoft Documents like Word, Excel, Powerpoint etc. Moreover TestNG and Apache POI don’t share any dependency with each other. Screenshot mechanism is nowhere dependent on TestNG and Apache POI
“import org.apache.commons.io.FileUtils;” is not being recognized in my program. Is there anything I would need to install?
Hi Kishan,
You can use FileHandler class. For more details, please check this link https://vistasadprojects.com/mukeshotwani-blogs-v2/how-to-use-filehandler-class-in-selenium-for-screenshot/
Hi Mukesh,
Can you please provide the tutorial for taking screenshot for failed test cases using Listeners.
Hi Shruti,
I’ll post it soon…:)
Hi Mukesh,
How can we capture screenshots for failed test cases only, by using iTestListener.
Hi Surajsingh,
Using ITestListner interface, it provides a method called onTestFailure() where you can call screenshot taking mechanism. This method will be called on whenever any test method fails.
hi..
can you tell us
about negative testing using selenium testNG
Hi Tejaswini,
Using dataProvider, provide wrong credentials for any application login and verify error message for each set of credentials. Please check this link https://vistasadprojects.com/mukeshotwani-blogs-v2/data-driven-framework-in-selenium-webdriver/ for better understanding. But I would suggest that negativce testing for UI is not recommendable.
Hi Tejaswini,
Using dataProvider, provide wrong credentials fro any application login and verify error message for each set of credentials. Please check this link https://vistasadprojects.com/mukeshotwani-blogs-v2/data-driven-framework-in-selenium-webdriver/ for better understanding
Hi Mukesh,
Just wanted to ask that how can we add and capture all screenshots for all credentials sent using data driven frame work in extent report.
Hi Shivam,
Just call ExtentTest object soon after passing credentials. Since credentials passing sequence will keep on repeating that much number of times screenshot along with ExtentTest statement will be called off.
Hi Mukesh…
Can we use @AfterTest instead of @AfterMethod.
i think it will work in both annotations.
Hi Amit,
This will work with AfterMethod only.
I am getting “java.lang.NullPointerException” while trying to take screenshot. Please help me on this.
Hi Sweta,
You are missing webdriver reference. Kindly check the code again.
Hi Mukesh,
I am able to make my code workable. I was initializing webdriver within @Test method as well which was giving me problem. Thank you for this video.
Great Sweta. Cheers
Hi Mukesh
How to take screen shots automatically when the test fails using JUnit?
Does JUnit support ITestResult interface?
Hi Rakesh,
I am not good in JUnit.
Hi Mukesh,
Hope you are well, Thanks for your videos and they are very helpful.
I am using jUnit framework, is there any interface equivalent to ITestResult in jUnit. Thanks
not sure on Soumya. You can switch to TestNG from Junit its more useful.
Hi Mukesh,
I want to able to take screenshots of failed tests. I have a TestBase Class and a PageBase class. I called the captureScreenshot method in the TestBase class inside the @AfterMethod but it does not seem to work and throws a NullPointerException.
Although when I use the same method code on it’s own it works fine. Can you help please? I am using PageObject Design pattern and running my tests from a testng.xml file. Thanks a lot.
Hey,
It seems some driver passing issue but without code cant guess the answer. Can you email your code mukeshotwani@learn-automation.com
Hi mukesh sir, when I am taking screenshot, it is coming in different font…… could you please help me in this issue?
Hi Kanchana what do you mean by font? I did not get can you please explain.
Hi Mukesh
I ahve taken the screen shot of the failed test case and stored in a folder in C Drive. Now I want to attach this file in the reports but I want to generalize the method means for each test case it should check if it is failed or passed if failed it should attach the image of the failed test case.
Hi Abhinav,
Its upto your logic to build the same. I wil suggest you to use BaseClass and put the screenshot login in @AfterMethod
Hi Mukesh,
I know this is out of the topic, but I would like to ask if there is a way to generate the extent reports to be attached to the email body message
Hey jay kindly refer below post for email http://www.assertselenium.com/java/emailable-reports-for-selenium-scripts/
hi i have one problem how to record faliure selenium scripts tell me sir
HI Palani,
Here is your solution https://vistasadprojects.com/mukeshotwani-blogs-v2/how-to-capture-screenshot-for-failed-test-cases-in-selenium-webdriver/
Hey Mukesh,
You are not only the brilliant…. you are the Extra Brilliant. Means, what’s the way of your teaching!!!! You are the superb man…… Keep teaching….. Keep rocking.
Thanks Prashant 🙂 Keep in touch
Hai..bro..
I’m getting error while capturing screen shot at fail case in simple g-mail login functionality…
Hey Asif,
What error u r getting kindly provide code as well.
Thanks Mukesh learnt new thing today.
Cheers Nikhil 🙂 Keep learning.
Can the report still run if I compiled it as a jar file?
Yes jay it will work
Nice tutorial, but you got typo in ULR to
“Please refer official doc for ITestResult”
Correct url is: http://testng.org/javadocs/org/testng/ITestResult.html
Cheers! 🙂
Thanks Adrian
Nice code Mukesh , learned something new today.thank you!
Thanks Nikhil 🙂
Hi Mukesh I have implemented your and it is taking the screenshot but i have created 10 testcases and i am making 4th test case to fail but it is not moving to the next test case please help me.
HI Adarsh,
Ideally it should move to next code/testcase if any test fails.
Please cross check once again and let me know.
unable to see the screenshots folder
Hi Anoop,
Can u explain in brief?
Thanks for the tutorial, i wants to implement failed test screencapture for appium ios and android tests on real devices.
please guide me.
regards
sree
Hi Sree,
Same code will work for appium too. I have implemented in my org.
Thanks for this useful tutorial. It helped me a lot in enhancing my Framework.
Hi Amit,
Thanks Keep visting 🙂
Thank you for this tutorial, i helped me to create good report into my automation framework tool.
Hi Sonia,
Good to hear that Thanks 🙂 keep visiting.
Thanks for this great tutorial.
It helped me a lot!
But I have a problem:
I’ve created a class separated to my test class and I don’t know how can I
set the TestResult status on my test class.
Should I set this property on every test method on my test class?
Thanks a lot!
`Hi Vinicius Lara,
Sorry for late reply I was out of town.
I will explain you how we categorize our test.
1- Create java class which can contain multiple test
2- Create an xml file which will execute above test and run this xml
3- If you have multiple xml then you can run through cmd or batch file as well.
Hope its clear let me know if you need more details I will share the links too.
Thanks
Mukesh