How to Capture Screenshot in Selenium Webdriver and Use in Reporting
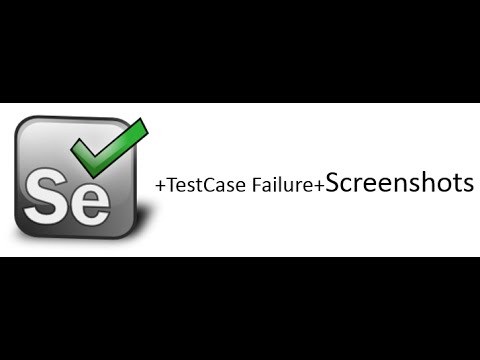
Hello, welcome to Selenium tutorials, in this post we will see how to capture Screenshot in Selenium Webdriver and use the same screenshot for reporting or for bug reporting or for any purpose.
In automation, it is mandatory to take the screenshot for verification so that it can prove also that your test case has covered certain functionality or not. Screenshots also help you a lot when your test case will fail so that you can identify what went wrong in your script or in your application.
Do you know that you can also use Robot class to capture screenshot in Selenium? When you capture screenshot using Robot Class it capture complete window screenshot. It will help you to capture alert as well which you can’t use with the normal screenshot method of Selenium.
Recently I used one of the reports called Extent Report which helps to attach the screenshot to the report which actually makes my reporting better than traditional TestNG reporting.
I also implemented screenshot on failure in my framework which makes my test more robust so I would recommend you to implement the same in your framework too.
Note- If you are using 3.6 and above version of Selenium then you should FileHandler Class in order to copy the screenshots.
I have created a youtube video on same.
Note-if you are working with 3.6 version and above
Recently Selenium has done some changes in recent version so if you are using Selenium 3.6.0 then you need to add below jar to project or if you are using then you can provide below dependency in project.
https://mvnrepository.com/artifact/commons-io/commons-io
How to capture Screenshot in Selenium webdriver
For taking screenshots Selenium has provided TakesScreenShot interface in this interface you can use getScreenshotAs method which will capture the entire screenshot in form of file then using FileUtils we can copy screenshots from one location to another location
Scenario – Open Google and take screenshot
Let’s implement the same
How to capture Screenshot in Selenium Webdriver
import java.io.File;
import java.io.IOException;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.Test;
public class ScreenshootGoogle {
@Test
public void TestJavaS1()
{
// Open Firefox
WebDriver driver=new FirefoxDriver();
// Maximize the window
driver.manage().window().maximize();
// Pass the url
driver.get("http://www.google.com");
// Take screenshot and store as a file format
File src= ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE);
try {
// now copy the screenshot to desired location using copyFile //method
FileUtils.copyFile(src, new File("C:/selenium/error.png"));
}
catch (IOException e)
{
System.out.println(e.getMessage());
}
}
If you use the above code then it will take the screenshot and will store in C:/selenium and screenshot name will be error.png
Note- If you are using Selenium version 3.6 and above then you will not find FileUtils class. You can use FileHandler class which is again part of Selenium. Please check detailed article on this.
Now consider a scenario where you have to take multiple screenshots then above code will be repetitive so for this we will create a small method which captures screenshots and will call this method from our script.
Example-
public static void captureScreenShot(WebDriver ldriver){
// Take screenshot and store as a file format
File src= ((TakesScreenshot)ldriver).getScreenshotAs(OutputType.FILE);
try {
// now copy the screenshot to desired location using copyFile method
FileUtils.copyFile(src, new File("C:/selenium/"+System.currentTimeMillis()+".png"));
}
catch (IOException e)
{
System.out.println(e.getMessage());
}
}
So this method we can call from our test case and call multiple time.
Copy the full code and try to execute in Eclipse
package com.bog.htmldemo;
The complete program for Screenshot in Selenium Webdriver
import java.io.File;
import java.io.IOException;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.Test;
public class ScreenshootGoogle {
@Test
public void TestJavaS1(){
// Open Firefox
WebDriver driver=new FirefoxDriver();
// call method
ScreenshootGoogle.captureScreenShot(driver);
// Maximize the window
driver.manage().window().maximize();
ScreenshootGoogle.captureScreenShot(driver);
// Pass the url
driver.get("http://www.google.com");
ScreenshootGoogle.captureScreenShot(driver);
}
public static void captureScreenShot(WebDriver ldriver){
// Take screenshot and store as a file format
File src=((TakesScreenshot)ldriver).getScreenshotAs(OutputType.FILE);
try {
// now copy the screenshot to desired location using copyFile method
FileUtils.copyFile(src, new File("C:/selenium/"+System.currentTimeMillis()+".png")); } catch (IOException e)
{
System.out.println(e.getMessage())
}
}
}
So in the above program, you can see I called captureScreenshot method three times like this you can create reusable actions in your script and you can use the same.