Fluentwait in selenium Webdriver and Different usage in Selenium
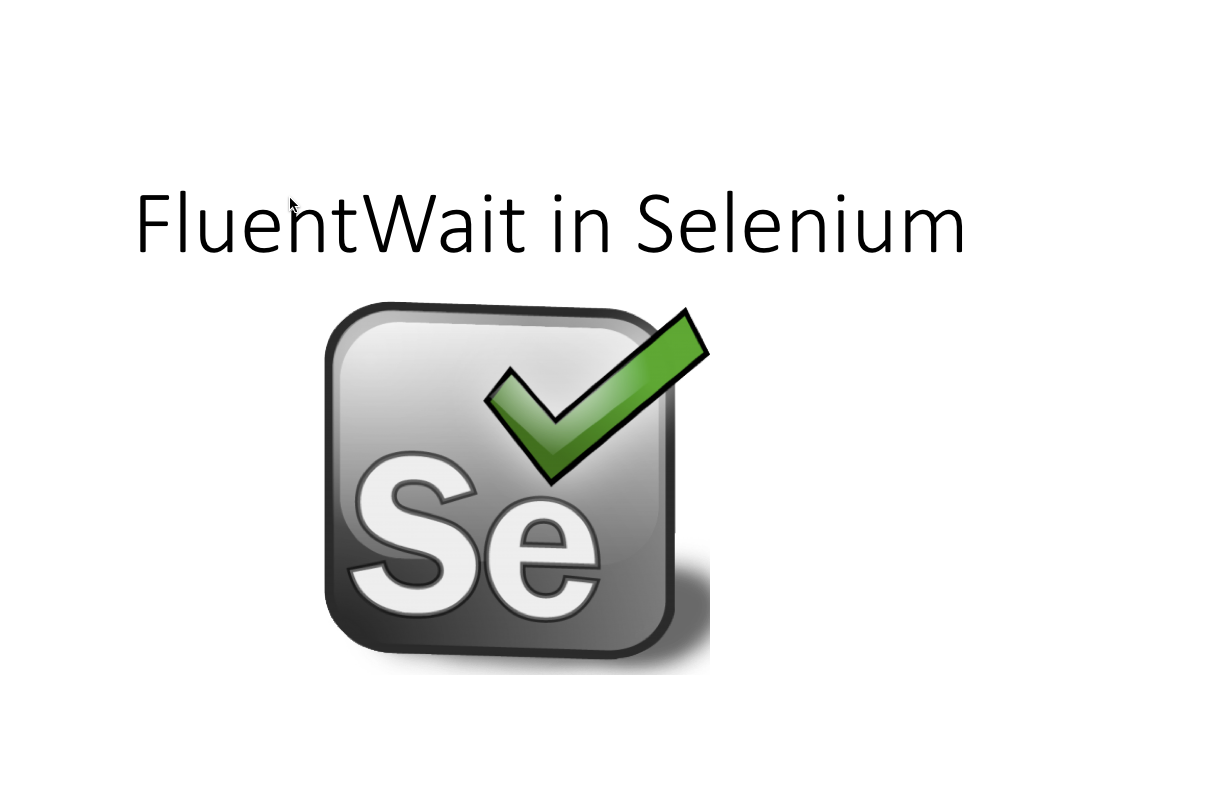
Wait in Selenium Webdriver is one of the most important topics for real-time applications and for interviews as well. Fluentwait in selenium webdriver in one of the examples of the dynamic wait which will solve many sync issues in Selenium Webdriver.
There are many kinds of wait available in Selenium which are
- Page Load timeout
- Implicit wait
- Explicit wait
- Fluent wait
Fluentwait in selenium webdriver is one of the most versatile wait which will give you the complete power to handle any kind of situation based on your requirement.
Why wait is required
1-Now a day many applications are using AJAX control, Jquery and many other complex widgets which make application slow and data takes some time to appear on screen so we need to wait until data is not present on UI.
2-When you perform the complex operation on any application it also takes some time for processing and we have to make sure our script should wait until specific conditions are not true.
There are much more examples in which we have to apply wait so that our script should not fail and we can get good ROI (Return of investment).
Definition of Fluentwait in selenium webdriver
In simple words, Fluent wait is just a plain class and using this class we can wait until specific conditions are met.
In Fluent wait, we can change the default polling period based on our requirement.
Technical details
1-Fluent wait is a class and is part of org.openqa.selenium.support.ui Package
2-It is an implementation of Wait interface.
3-Each fluent wait instance defines the maximum amount of time to wait for a condition and we can give the frequency with which to check the condition.
4- We can also ignore any exception while polling element such as NoSuchElement exception in Selenium.
Now we have discussed enough on Fluent wait and its usage so let’s talk about the implementation part of it and how we can use in our project or in our framework.
You will also get questions in your interviews that what is the difference between explicit wait and Fluent wait.
Ans- In explicit wait, you can use some set of existing precondition to wait like
Wait till element is not visible, wait till element is not clickable, Wait till presence of element located and so on.
In Fluent wait, you can customize the apply method and you can write your own conditions based on your requirement.
Note- 95 % of your test can be handled via explicit wait and in some cases, you have to use Fluent wait so it totally up to you.
Syntax of Fluentwait in selenium webdriver
// Waiting 60 seconds for an element to be present on the page, checking
// for its presence once every 2 seconds.
Wait<WebDriver> wait = new FluentWait<WebDriver>(driver) .withTimeout(60, SECONDS) .pollingEvery(2, SECONDS) .ignoring(NoSuchElementException.class); WebElement foo = wait.until(new Function<WebDriver, WebElement>() { public WebElement apply(WebDriver driver) { return driver.findElement(By.id("foo")); } });
As you can see the syntax is quite complex but we don’t have to worry about that because we have to change only Apply method which will make our task easy.
FluentWait in Selenium Webdriver
Requirement-
1- Click on timer
2- Wait till the WebDriver text is not coming.
3- Once WebDriver appears- Stop the wait and print the status.
Complete program of Fluentwait in selenium webdriver
package class10; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.support.ui.FluentWait; import com.google.common.base.Function; public class FluentWaitDEmo { public static void main(String[] args) throws InterruptedException { // Start browser WebDriver driver = new ChromeDriver(); // Maximize browser driver.manage().window().maximize(); // Start the application driver.get("http://seleniumpractise.blogspot.in/2016/08/how-to-use-explicit-wait-in-selenium.html"); // Click on timer so clock will start driver.findElement(By.xpath("//button[text()='Click me to start timer']")).click(); // Create object of FluentWait class and pass webdriver as input FluentWait<WebDriver> wait = new FluentWait<WebDriver>(driver); // It should poll webelement after every single second wait.pollingEvery(1, TimeUnit.SECONDS); // Max time for wait- If conditions are not met within this time frame then it will fail the script wait.withTimeout(10, TimeUnit.SECONDS); // we are creating Function here which accept webdriver and output as WebElement- WebElement element = wait.until(new Function<WebDriver, WebElement>() { // apply method- which accept webdriver as input @Override public WebElement apply(WebDriver arg0) { // find the element WebElement ele = arg0.findElement(By.xpath("//p[@id='demo']")); // Will capture the inner Text and will compare will WebDriver // If condition is true then it will return the element and wait will be over if (ele.getAttribute("innerHTML").equalsIgnoreCase("WebDriver")) { System.out.println("Value is >>> " + ele.getAttribute("innerHTML")); return ele; } // If condition is not true then it will return null and it will keep checking until condition is not true else { System.out.println("Value is >>> " + ele.getAttribute("innerHTML")); return null; } } }); // If element is found then it will display the status System.out.println("Final visible status is >>>>> " + element.isDisplayed()); } }
Final thoughts- The same requirement can be fulfilled via explicit wait so its up to you which one to prefer. I would recommend you to create a library for this and you can use whenever it’s required.
In my org, we have created a custom library which takes care of Sync issue and we have to use less explicit wait and fluent wait of Selenium Webdriver.
You can try this wait with multiple scenarios and it’s up to you. In case if you have any thoughts related to this then don’t hesitate to share. Let me know the same in the comment section and if you find this article helpful then feel free to share with your friends as well.
Hi Mukesh,
I only see one use of fluent wait that we can ignore exception of our choice.
But is there any exception that can occur before nosuchelement exception in any condition that we need to be vigilant about?
E.g. Exception A can occur before nosuchelement exception so give it while polling and Explicit only handles nosuchelement exception so we have to use fluent.
Please correct if I have quoted anything wrong.
Regards
Anuj Kapur
Hi Anuj,
Please visit https://www.selenium.dev/selenium/docs/api/java/org/openqa/selenium/package-tree.html and have look at tree java.lang.Exception -> java.lang.RuntimeException -> org.openqa.selenium.WebDriverException
Thanks Mukesh 🙂
Hi Mukesh
Can we implement a common method for fluent wait so that we can put in some base class and can pass web element dynamically.
Hi Sulabh,
Usually base class doesn’t deal with WebElements as these are handled in Page Classes. So under any utility class, you create a method which accepts WebElements as parameter and process Fluent Wait and returns same WebElement itself
Thanks Mukesh
Actually my mean was util class its just my misunderstanding.
yes…:)
How to wait for page load in selenium?
Hi Ancia,
By default, Selenium will wait for page load. You can also set the max page load timeout in Selenium.
Hi Mukesh,
Im not able to understand what is the difference between xpath used in overrided apply() method and normal apply() method.
The xpath you have used refers to same element i.e. WebDriver whihc is default one
.//p[@id=”demo”] in overrided function
//p[text(),”WebDriver”] original apply function denotes the same element.
My question is why cannot we use the same xpath of //p[text(),”WebDriver”] in overrided apply method and capture its inner text?
Hi Rabiya,
Both xpath behaves in same manner. One used id and another used text. But when we verify any textual content on screen then usually it is recommendable to use xpath which itself contains same text. You can use //p[@id=’demo’]
Hi Mukesh,
I could not find any scenario where in FluentWait can help but WebDriver wait can not .
It would be great if you can elaborate on one such example to clear my confusion between the two waits.
Hi Prakrati,
I agree WebdriverWait has already many preconditions which can serve our purpose. FluentWait can help you to customize your condition.
Hi Prakarati,
One such scenario which I came across where it was mandatory to use Fluent wait was:
“There was a text field on the page which took dynamic time to have a value populated. Also the value on that text field didn’t appear AJAX style, we had to click on a refresh button in the page(not the page refresh) to check if the field has a value.
We had to keep checking this for upto 5 minutes.”
hi mukesh
iam very intersting to learn selenium webdriver .please suggest which topic is started
Hi Veluri,
You can start from here https://vistasadprojects.com/mukeshotwani-blogs-v2/testng-tutorials-for-beginners/ and go through of these one by one
Hi Mukesh everytime I run my code I get StateElementReferenceException. I added wait command to my code, then I get TimeoutException.
Can you help me out on this.
Hi Priyanka,
May be this can help you https://vistasadprojects.com/mukeshotwani-blogs-v2/how-to-solve-stale-element-reference-exception-in-selenium-webdriver/
Hi Mukesh,
can u please help me to get the sample program syntax for FluentWait class.
please note that, i am getting class name in the hint, but it is not displaying the syntax details as u mentioned in the video and not only for this class but for all the other hints as well.
could you please help me if any setting is needed for this functionality?
thanks in advance
Hi Vinay,
You have to attach source for this then only you will get the documentation.
Thank you very much for your course
Thanks Masi
Hi Mukesh,
In my program ,I am getting two errors.
kindly kelp to resolve.
1.Multiple markers at this line
– The type FluentWait is not generic; it cannot be parameterized with arguments
2.The import org.openqa.selenium.support.ui.FluentWait conflicts with a type defined in the same file
I have copy pasted the above code and using Selenium 3.But still getting above mentioned errors
Thanks
HI Bobby,
I just tried and worked. Can you check your code again.
I would suggest you to watch video and write code from your side which will make your hand dirty and you will learn quickly.
In my org, we have created a custom library which takes care of Sync issue and we have to use less explicit wait and fluent wait of Selenium Webdriver.
Shiak Subhani : Hi Mukesh, I am a big fan of your blog, it’s really good to new learners. I have some doubts in this article. Please reply me asap.
How can we create custom library ? Could you please send reference video link ? It would be good, if you share video link. Thanks in advance.
Hi Subhani,
I am really glad to know that you liked blog and YouTube videos.
Here is the sample code for custom lib which i was talking about.
https://vistasadprojects.com/mukeshotwani-blogs-v2/best-way-to-handle-synchronization-in-selenium-webdriver/