Using listener Re run failed test cases in selenium automatically
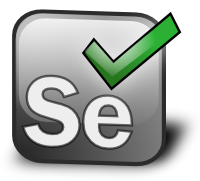
In this article, we are going to use new Interfaces today which will allow you to Re run failed test cases in selenium if the test fails.
I have used this multiple times in my scripts and its helps me a lot. Hope this will be helpful for you as well.
Why Re run failed test cases in selenium if it is failing?
While working with internal applications, you may notice that your test is failing unnecessarily at different point and once you re-run the same test it passes without any changes
There are multiple reasons why the test fails.
- Due to the network issue.
- Due to application downtime.
- Due to loading issue and etc.
But if the script is failing due to xpath and some valid reason then you have to maintain for re work on your scripts.
This is one of the interview questions as well so make sure you are able to implement below scenario.
I have already updated 100 Selenium Interview question so if you are looking for job change then have a look.
100 Selenium Webdriver interview questions.
What or how we Re run failed test cases in selenium in case of Failure?
It sounds very tough to re-run scripts automatically but it is not. We need to write separate JAVA class that will take care of running your test if failure.
We will be using one IRetryAnalyer interface that is part of TestNG and we need to override retry method.
Read official documentation about IRetryAnalyzer
Create separate Class which will implement IRetryAnalyer interface
package TestNGDemo; import org.testng.IRetryAnalyzer; import org.testng.ITestResult; // implement IRetryAnalyzer interface public class Retry implements IRetryAnalyzer{ // set counter to 0 int minretryCount=0; // set maxcounter value this will execute our test 3 times int maxretryCount=2; // override retry Method public boolean retry(ITestResult result) { // this will run until max count completes if test pass within this frame it will come out of for loop if(minretryCount<=maxretryCount) { // print the test name for log purpose System.out.println("Following test is failing===="+result.getName()); // print the counter value System.out.println("Retrying the test Count is=== "+ (minretryCount+1)); // increment counter each time by 1 minretryCount++; return true; } return false; } }
Now we are done almost only we need to specify this in the test case.
@Test(retryAnalyzer=Retry.class)
In above statement, we are giving instruction to our test case that if the test case fails then it will call Retry class that we created above.
Program to Re run failed test cases in selenium
import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.testng.Assert; import org.testng.annotations.Test; public class VerifyTitle { // Here we have to specify the class – In our case class name is Retry @Test(retryAnalyzer=Retry.class) public void verifySeleniumTitle() { WebDriver driver=new FirefoxDriver(); driver.manage().window().maximize(); driver.get("https://vistasadprojects.com/mukeshotwani-blogs-v2"); // Here we are verifying that title contains QTP or not. This test will fail because title does not contain QTP Assert.assertTrue(driver.getTitle().contains("QTP")); } }
Console-
When you run above program you will notice that our test will run 4 times.
The first time it will run normally and three times more due to Retry class that we have written.
Hope this article will help. Please comment below if any issue or doubt in this article.
Have a nice day 🙂
Thanks a lot!!!! you really save my life. I have to execute same testscript for 500 times and manually i was running the script. Now it will run automatically …………
Great, Aparna…:)
Hi Mukesh, Do we need to mentioned “retryAnalyzer=class name” for each test case. If there are many test cases let say more that 100, then how should we handle this?
Thanks in advance
Hi Ashish,
The one which I mentioned was easiest way but will be difficult to use in your scenario. You can create a class which should implements IAnnotationTransformer interface and here you can deal use same Retry.class and add this former class as listener to testng.xml file.
Hi Mukesh can you please explain this by giving an example.
Thanks in advance.
Hi Anuj,
I’ll do it soon…:)
Hi Mukhesh, The above information is very helpful, It’s the code for automatic run whenever test got failed? Or we need to click again any XML file to run
Hi Nasreen,
This will automatically trigger failed test scripts
Hi Mukesh
Please upload keydriven framework video in selenium
Hi Sandeep,
I’ll publish it soon.
Hi Mukesh,
I m using mac system to run the automation script. I am unable to upload the images through mac. In my application , needs to click on Choose image file button —> Upload image button —> then needs to be upload the image from the folder. Please help me to solve this issue.
Hi Jagadeesh,
This will help https://vistasadprojects.com/mukeshotwani-blogs-v2/upload-file-in-selenium-in-mac/
Hi Mukesh,
our Tutorials are very helpful. I have one doubt. The code which you have written will retry 4 times . will this code exit the loop if your program doesn’t want to go for a retry. Can you explain where we have pointed out that.
Why are sending False as it comes out of If loop. So always we return False why??
Hi Nikhil,
Once total count is done we have to come out of the loop so we used false.
Thanks Mukesh,it’s useful alot for me,but i want to apply this retryAnalyzer to Specific testcases,so please give me the ans.
Thanks.
Hi Naresh,
Use
@Test(retryAnalyzer=Retry.class) to run specific test.
Thanks Mukesh.
Your most welcome Naresh.
Hi….
this article is useful and how to record videos only failed test case while rerun that same test case
Hi Anu,
For video you need to add another jar Monte. Kindly check other blogs where they already uploaded for the same. I will also created article on this soon.
Hi Mukesh,
Your site is very good. Please let me know the video’s link,so that i can learn more .
HI ,
I like this site and the way of discussion . I am waiting for Video on this Re-run .
Thanks In advance.
Thanks Shaik, will upload video soon.
For some test its working but some test its not working getting “Error 500–Internal Server Error”
Hi Brijendra,
500 it is related to application only so try to report to the developer.
Hi Mukesh Thanks 🙂
I have used above link and now I am able to rerun the failed test cases. Thanks
One More Query : If any of the test case (@test) failed I will get the emailable- report as failed(Failed due to network issue ). But The same test case is getting passed in the retry.
In this case I want the Final Report as Passed. How to achieve this Scenario ?
Hi Kiran,
It should display pass only let me check and will update you
is there any way to implement in suite level rather than test level.. suppose I have 4000 tc. i can not go each test case and apply this .. rather than if u have any thing on suite level.. please let me know.
Hi Priya,
Very nice question, yes it is possible. I will create post on this and will update soon.
I can suggest You can create listener for this and specify in the testng.xml and it will be applicable for all tests.
Thanks
Mukesh
Hi Mukesh,
This article is very helpful, but again can you please show us how to run this at suit level for all the test cases? Thank you.
Regards,
Sai Chandra.
Hi Sai,
Will update by sunday 🙂
Nice One ! If we get a Video for this it will be great
Thanks Kiran,
On the way will upload on Saturday..
Hi Mukesh ,
Ur site is very helpful to understand all concepts easily ,
but ,I tried Re- Run is not happening for me.please guide me.
Thanks in advance.
Regards ,
Apoorva
Hi Apoorva,
I will create video on the same and will update you.