How to get current system date and time in Java/ Selenium
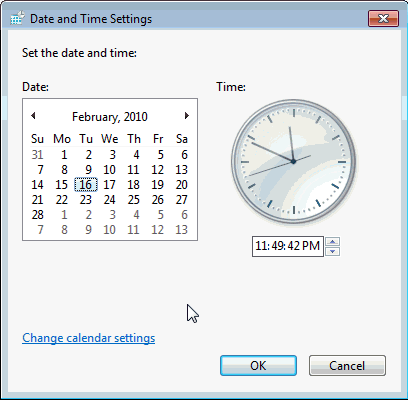
Hello Guys, Welcome back to Selenium tutorial today we will see some new topic which might help you in some cases where you have to handle with dates. Today I will share How to get current system date and time in Java/ Selenium so that you can verify the same in your test cases.
How to get current system date and time in Java/ Selenium
Today I was automating some of my test cases and I got one requirement where I have to validate some record with date and time. In this case I captured date and time and verified respectively.
Step which I have taken
1- Decide which date format you want, then we can use SimpleDateFormat class to do the same. I took below format
SimpleDateFormat(“MM/dd/yyyy”)
2- Create Date class object which will return Date and time
3- Then using format method get the date and time.
Program 1- Write a program which will capture System Date
package demo; import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.Date; public class GetDateinJava { public static void main(String[] args) { // Create object of SimpleDateFormat class and decide the format DateFormat dateFormat = new SimpleDateFormat("MM/dd/yyyy "); //get current date time with Date() Date date = new Date(); // Now format the date String date1= dateFormat.format(date); // Print the Date System.out.println(date1); } }
OUTPUT
Program 2- Write a program which will capture System Date/ Time
In this example I am taking time as well so I have added HH:MM:SS also in format
package demo; import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.Date; public class GetDateinJava { public static void main(String[] args) { // Create object of SimpleDateFormat class and decide the format DateFormat dateFormat = new SimpleDateFormat("MM/dd/yyyy HH:mm:ss"); //get current date time with Date() Date date = new Date(); // Now format the date String date1= dateFormat.format(date); // Print the Date System.out.println("Current date and time is " +date1); } }
OUTPUT
Program 3- Write a program which will capture Only Current date
import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.Date; public class DateExample { public static void main(String[] args) { // here we are only capturing current date, which you can use for your automated scripts. DateFormat dateFormat = new SimpleDateFormat("dd"); //get current date time with Date() Date date = new Date(); // Now format the date String date1= dateFormat.format(date); System.out.println(date1); } }
Output
I hope now you are comfortable with Dates now. Let me know if you are facing any issues.
For More updates Learn Automation page
For any query join Selenium group- Selenium Group
Hi Mukesh,
I need your help with the date and time assertion. My assertion is passing in local but in Travis, it’s failing, and I am seeing the difference of 5 and half hours
[2022-10-17 15:13:29] [+0000] [INFO] [org.junit.ComparisonFailure: expected: but was:]
In Travis, the timezone is – export TZ=America/New_York. So I tried in many ways setting lang=en-us but did not work. Could you please let me know if there is any solution for this time comparision
Hi Devi, I have not worked on travis CI but hope below thread will work https://stackoverflow.com/questions/23371542/how-do-i-configure-travis-ci-to-use-the-correct-time-zone-for-a-rails-app
How to capture current date and time of a country of City?
Hi Priya,
For this, you need to use some APIs that provide data. As of now, I don’t have ready code for this. Please check this link https://stackoverflow.com/questions/55901/web-service-current-time-zone-for-a-city
Hello sir, I am taking multiple inputs from excel sheet and running a test case . If 2 or more input tests fail then also this will create single screenshot returns the same name on every failure so this will create a single screenshot every time due to the same name. Please tell me how to set a different name to every failure test screenshot?
Hi Beula,
While naming screenshot file name, use some dynamic name over there like current System date time(including minutes and seconds).
Example: methodWhichReturnsCurrentDateTime+”.png”
Hi,
How to select date when it is dynamically changing every day
for any sites when we use for transportation booking
how to select current date from calendar
and another date for return booking
Hi Rajesh,
Below post will guide you for the same.
https://vistasadprojects.com/mukeshotwani-blogs-v2/selenium-advance-activity/
how to print current date and time in the emailable-report.html….can u give any suggestions???
Hi Minnnu,
Kindly use below report learn-automation.com/advance-selenium-reporting-with-screenshots-2/
Hi mukesh, I am using the same method to check the current date in my application. however the test cases are failed if they are executed by my onsite folks(USA).
Hey Mukesh,
What error or exception it is throwing?
Thank you very much it worked for me.
hello sir, using selenium can we run a script at a particular time of a day or on a regular basis. Suppose I have to send an email or a message to one of my friend at 2pm, and I had already written the message in a selenium script and i want that message to be send at that time of day only
Yes manoj using jenkins we can do that https://vistasadprojects.com/mukeshotwani-blogs-v2/selenium-integration-with-jenkins/
Hi, make sure you are using correct letter pattern.
mm is not the same as MM.
m – Minute in hour
M – Month in year
ref.: https://docs.oracle.com/javase/7/docs/api/java/text/SimpleDateFormat.html
Hope it will helped 😉
Al
Thanks Algirdas
Hi Mukesh,
While executing my program, the month is changing repetitively for every run of the program.The output should be 2016/03/10.
But the result is ,
First run output1: 2016/29/10
Second run output2: 2016/32/10
Can u tell me which it is changing ??
Thanks in advance.
Hi Mahesh,
not sure I will check and let u know.