Handle Dropdown in Selenium with C# and Handle Multiple Values
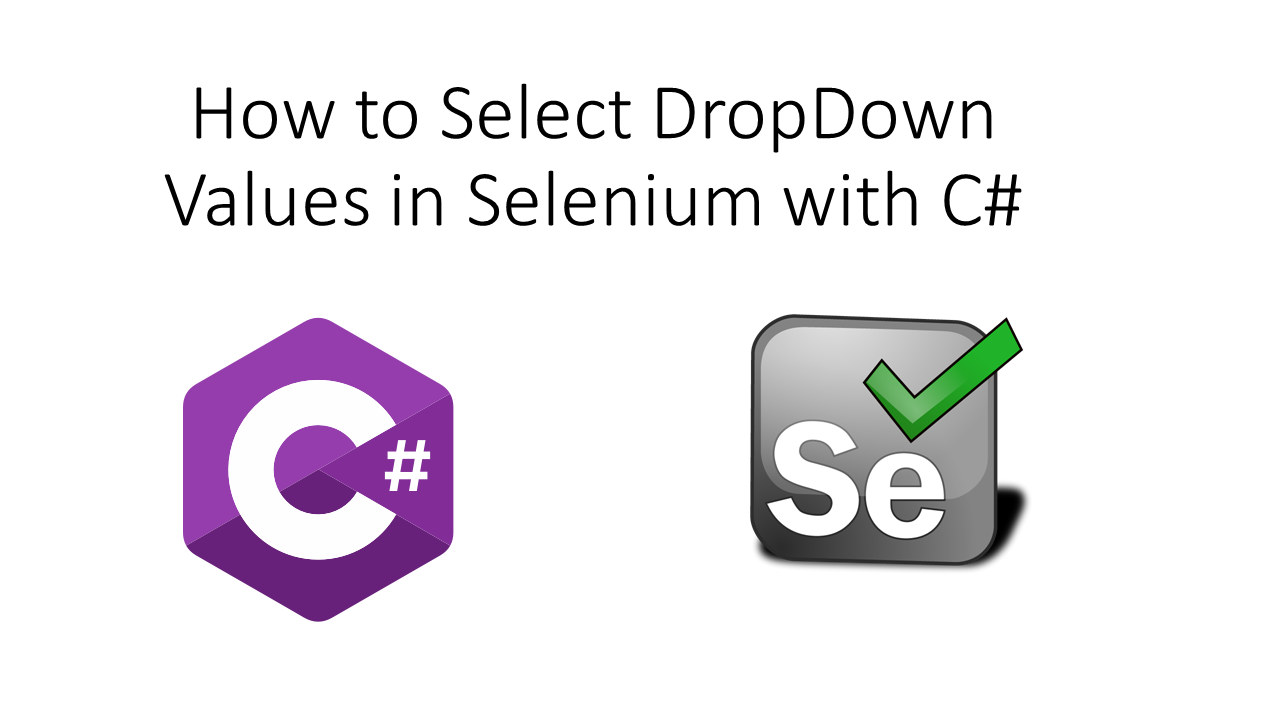
The dropdown is like a normal WebElement for Selenium but when it comes to handling it like Selecting the value, deselecting the value or capture some values out of it.
Those who are new in HTML for them – Dropdown is a method of showing a large list of choices, but only one choice is displayed initially until the user select/ click on the drop down. A dropdown can be used to select between choices in a form.
In our previous post, we have seen how to deal with normal web elements like textbox, buttons etc
If you are completely new to Selenium then you can get all information about installation using this link.
Handle Dropdown in Selenium Webdriver with C#
Before we start I would recommend you to check Official Documentation of SelectElement Class which will give you a clear idea about what we are planning to do.
In C# we can select the dropdown elements by using SelectElement class.
First, we should create an object for SelectElement class by passing the dropdown element as parameter.
Different Select operations for Dropdown
We can select the dropdown elements using different methods.
SelectByText – This method selects the option given under any dropdown by using the text displayed on the element. This method takes a string value to be selected as an argument and return type is void.
Syntax – void SelectElement.SelectByText(string text, [bool partialMatch = false])
We can also use this method for partial match text selection by passing 2nd argument as true.
SelectByIndex – This method selects the option based on the index of the element. This method takes index of the element in the list as an argument and return type is void.
Syntax – void SelectElement.SelectByIndex(int index)
SelectByValue – This method selects the option based on the value of the element. This method takes value of the element as an argument and return type is void.
Syntax – void SelectElement.SelectByValue(string value)
Other operations Apart from Selecting values
Options – We can get the list of all options of the select element.
Syntax – IList<IWebElement> SelectElement.Options { get; }
isMultiple – This method will tell us whether the element supports multiple selections or not.
Syntax – boolean SelectElement.IsMultiple { get; }
These methods only work for multi-select elements not for dropdown elements.
DeselectAll – Deselect all selected elements. This method does not require any argument and return type is void.
Syntax – void SelectElement.DeselectAll()
DeselectByIndex – Deselect the option at given index. This method takes index as argument and return type is void.
Syntax – void SelectElement.DeselectByIndex(int index)
DeselectByText – Deselect all the options, with the same text as argument. This method takes text as argument and return type is void.
Syntax – void SelectElement.DeselectByText(string text)
DeselectByValue – Deselect all the options, with the same value as argument. This method takes value as argument and return type is void.
Syntax – void SelectElement.DeselectByValue(string value)
Example
Open Chrome and start Facebook
Select any date of birth
Check dropdown is multi Select
Print all values of Month Dropdown
// Create a driver instance for chromedriver IWebDriver driver = new ChromeDriver(); //Navigate to google page driver.Navigate().GoToUrl("https://www.facebook.com"); //Maximize the window driver.Manage().Window.Maximize(); //Find the dropdown using xpath IWebElement element_xpath = driver.FindElement(By.XPath("//*[@aria-label='Month']")); //Create a select object SelectElement selectMonth = new SelectElement(element_xpath); //select the option by text. selectMonth.SelectByText("Jun"); //Find the dropdown using id IWebElement element_id = driver.FindElement(By.Id("day")); //create a select object SelectElement selectDay = new SelectElement(element_id); //Select the option by index. selectDay.SelectByIndex(4); //Find the dropdown using name IWebElement element_name = driver.FindElement(By.Name("birthday_year")); //create a select object SelectElement selectYear = new SelectElement(element_name); //Select the option by value. selectYear.SelectByValue("1990"); //Options of Month IList<IWebElement> elements = selectMonth.Options; //Get the count of options int elementsSize = elements.Count; //Print all elements for(int i=0; i<elementsSize;i++) { Console.WriteLine("Value at "+ i +" is: "+elements.ElementAt(i).Text); } //Check if day is multiple selection or not. Boolean boolValue = selectDay.IsMultiple; //print the result Console.WriteLine("Day is Multiple selection :"+boolValue); //close the driver driver.Close();
The output of the program is
I hope you liked above article, if yes then please share with your friends and team mates.
Hi Mukesh,
Can you share link for handling dropdown with tags other than select.
Hi Ligiya,
Please refer this link https://vistasadprojects.com/mukeshotwani-blogs-v2/handle-bootstrap-dropdown-in-selenium-webdriver/
Mukesh Sir,
I don’t have any experience , Please help with the interview questions that I’m not able to access your audio video. I really need it . I don’t know what to answer in interview Please save me. Thanks in Advance Sir!
Hi Vaidehi,
Please refer this link https://vistasadprojects.com/mukeshotwani-blogs-v2/selenium-interview-questions-and-answers/